The Java ArrayList function is a class that provides a dynamic array implementation in Java. It allows for the creation of resizable arrays that can be modified during runtime. The ArrayList class provides methods to add, remove, and access elements in the array. It also provides methods to search for elements, sort the array, and perform other operations. The ArrayList class is part of the Java Collections Framework and is commonly used in Java programming for its flexibility and ease of use. Keep reading below to learn how to Java ArrayList in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
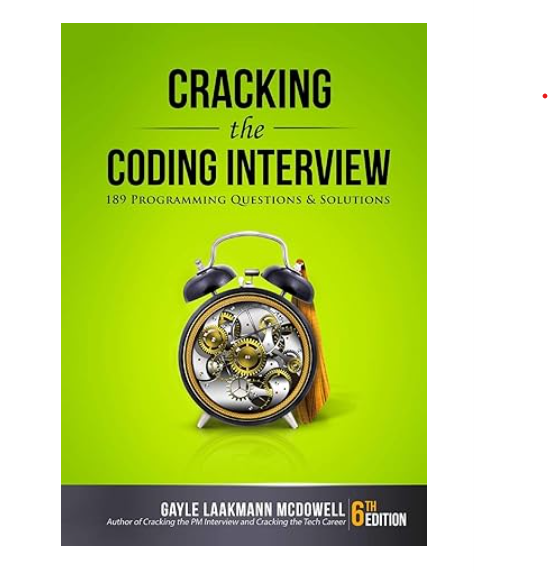
Java ArrayList in C++ With Example Code
Java ArrayList is a dynamic array that can be used to store elements of any data type. It is a part of the Java Collection Framework and provides many useful methods to manipulate the elements in the list. In this blog post, we will explore how to implement a similar data structure in C++.
To implement an ArrayList in C++, we can use a vector. A vector is a dynamic array that can be resized during runtime. It is a part of the Standard Template Library (STL) and provides many useful methods to manipulate the elements in the vector.
To create a vector in C++, we need to include the
#include
using namespace std;
int main() {
vector
return 0;
}
In the above code, we have created an empty vector of integers named “myVector”. We can add elements to this vector using the push_back() method. Here is an example code to add elements to the vector:
#include
using namespace std;
int main() {
vector
myVector.push_back(10);
myVector.push_back(20);
myVector.push_back(30);
return 0;
}
In the above code, we have added three elements (10, 20, and 30) to the vector using the push_back() method. We can access the elements in the vector using the [] operator. Here is an example code to access the elements in the vector:
#include
#include
using namespace std;
int main() {
vector
myVector.push_back(10);
myVector.push_back(20);
myVector.push_back(30);
cout << myVector[0] << endl; // Output: 10
cout << myVector[1] << endl; // Output: 20
cout << myVector[2] << endl; // Output: 30
return 0;
}
In the above code, we have accessed the elements in the vector using the [] operator and printed them to the console.
In conclusion, we can implement a Java ArrayList in C++ using a vector. A vector is a dynamic array that provides many useful methods to manipulate the elements in the vector. We can create a vector, add elements to it, and access the elements using the [] operator.
Equivalent of Java ArrayList in C++
In conclusion, the equivalent function to Java's ArrayList in C++ is the std::vector. Both data structures provide dynamic resizing and random access to elements. However, there are some differences in syntax and functionality between the two. For example, Java's ArrayList has built-in methods for adding, removing, and sorting elements, while C++'s std::vector requires the use of algorithms or manual manipulation. Despite these differences, both Java's ArrayList and C++'s std::vector are powerful tools for managing collections of data in their respective languages. As a programmer, it's important to understand the similarities and differences between these data structures in order to choose the best one for your specific needs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |