The Java ArrayList function is a class that provides a dynamic array implementation in Java. It allows for the creation of resizable arrays that can be modified during runtime. The ArrayList class provides methods to add, remove, and access elements in the array. It also provides methods to search for elements, sort the array, and perform other operations. The ArrayList class is part of the Java Collections Framework and is commonly used in Java programming for its flexibility and ease of use. Keep reading below to learn how to Java ArrayList in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
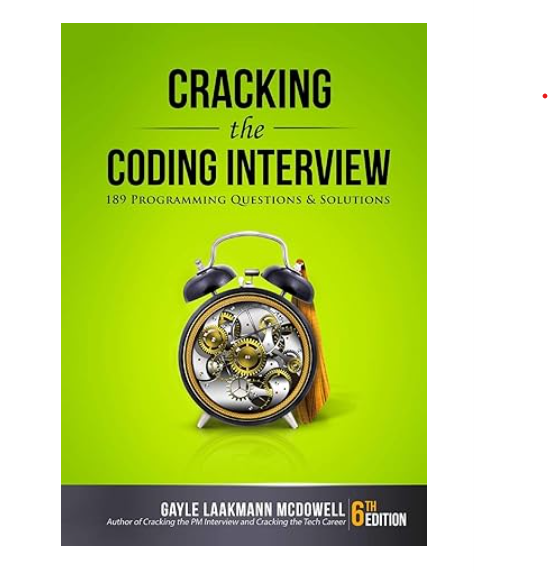
Java ArrayList in Go With Example Code
Java developers who are transitioning to Go may be wondering how to implement the Java ArrayList in Go. In Java, ArrayList is a dynamic array that can grow or shrink as needed. In Go, slices are the equivalent of dynamic arrays.
To create a slice in Go, you can use the built-in `make` function. Here’s an example:
mySlice := make([]int, 0)
This creates an empty slice of integers. You can add elements to the slice using the `append` function:
mySlice = append(mySlice, 1)
This adds the integer 1 to the end of the slice. You can also add multiple elements at once:
mySlice = append(mySlice, 2, 3, 4)
This adds the integers 2, 3, and 4 to the end of the slice.
To access elements in the slice, you can use the index notation:
fmt.Println(mySlice[0]) // prints 1
This prints the first element in the slice, which is 1.
To remove elements from the slice, you can use the `append` function again, but with a slice of the elements you want to keep:
mySlice = append(mySlice[:2], mySlice[3:]...)
This removes the third element from the slice (which has an index of 2 in Go).
In summary, Go’s slices are the equivalent of Java’s ArrayLists. You can create a slice using the `make` function, add elements using the `append` function, access elements using the index notation, and remove elements using the `append` function with a slice of the elements you want to keep.
Equivalent of Java ArrayList in Go
In conclusion, Go provides a powerful and efficient way to manage collections of data through its built-in slice type. While it may not have an exact equivalent to Java’s ArrayList function, Go’s slice type offers similar functionality and more flexibility. With its ability to dynamically resize and manipulate elements, Go’s slice type is a great choice for managing collections of data in a variety of applications. Whether you’re a seasoned Java developer or new to Go, understanding the similarities and differences between these two languages can help you make informed decisions about which tools to use for your next project.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |