The Java ArrayList function is a class that provides a dynamic array implementation in Java. It allows for the creation of resizable arrays that can be modified during runtime. The ArrayList class provides methods to add, remove, and access elements in the array. It also provides methods to search for elements, sort the array, and perform other operations. The ArrayList class is part of the Java Collections Framework and is commonly used in Java programming for its flexibility and ease of use. Keep reading below to learn how to Java ArrayList in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
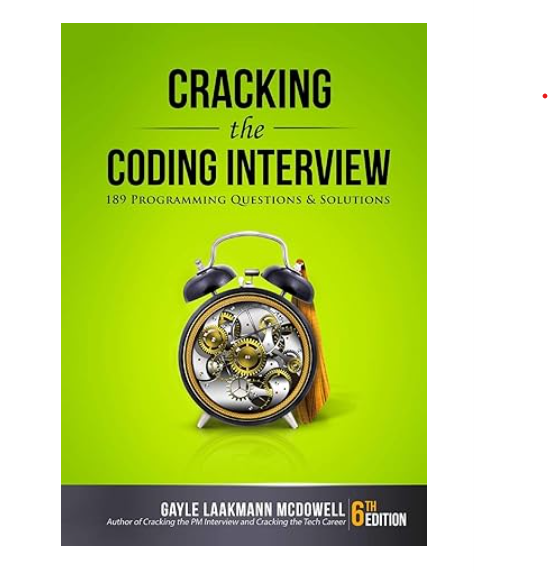
Java ArrayList in Rust With Example Code
Java ArrayList is a popular data structure used in Java programming language. It is a dynamic array that can grow or shrink in size as per the requirement. Rust is a modern programming language that is gaining popularity due to its performance and safety features. In this blog post, we will discuss how to implement Java ArrayList in Rust.
To implement Java ArrayList in Rust, we need to first understand the basic concepts of ArrayList in Java. ArrayList is a resizable array that can store elements of any data type. It is implemented using an array and provides methods to add, remove, and access elements from the array.
In Rust, we can implement ArrayList using the Vec data structure. Vec is a dynamic array that can grow or shrink in size as per the requirement. It is implemented using a vector and provides methods to add, remove, and access elements from the vector.
Let’s take a look at an example code to implement ArrayList in Rust using Vec:
fn main() {
let mut arr: Vec
arr.push(10);
arr.push(20);
arr.push(30);
println!("{:?}", arr);
}
In the above code, we have created a new Vec of type i32 and added three elements to it using the push method. We have then printed the contents of the Vec using the println macro.
We can also use the remove method to remove elements from the Vec. Let’s take a look at an example code to remove an element from the Vec:
fn main() {
let mut arr: Vec
arr.remove(1);
println!("{:?}", arr);
}
In the above code, we have created a new Vec of type i32 and added three elements to it using the vec! macro. We have then removed the element at index 1 using the remove method and printed the contents of the Vec using the println macro.
In conclusion, we can implement Java ArrayList in Rust using the Vec data structure. Vec provides similar methods to add, remove, and access elements from the array as ArrayList in Java. Rust’s safety features and performance make it a great choice for implementing data structures like ArrayList.
Equivalent of Java ArrayList in Rust
In conclusion, Rust’s Vec
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |