The Java ArrayList function is a class that provides a dynamic array implementation in Java. It allows for the creation of resizable arrays that can be modified during runtime. The ArrayList class provides methods to add, remove, and access elements in the array. It also provides methods to search for elements, sort the array, and perform other operations. The ArrayList class is part of the Java Collections Framework and is commonly used in Java programming for its flexibility and ease of use. Keep reading below to learn how to Java ArrayList in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
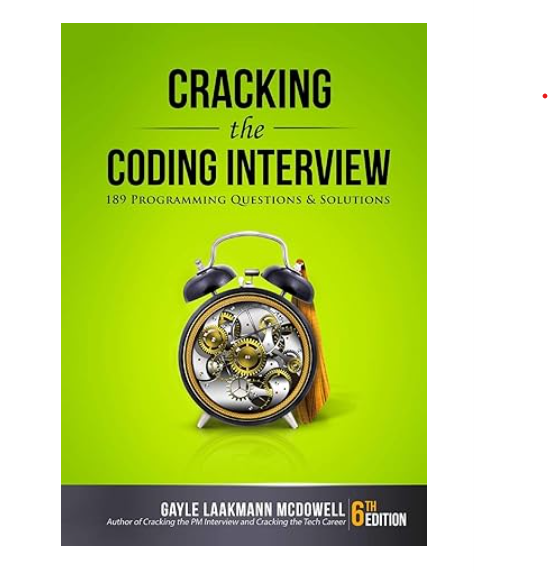
Java ArrayList in TypeScript With Example Code
Java ArrayList is a popular data structure used in Java programming. However, if you are working with TypeScript, you may wonder how to use ArrayList in TypeScript. In this blog post, we will explore how to use Java ArrayList in TypeScript.
First, let’s understand what ArrayList is. ArrayList is a resizable array implementation of the List interface in Java. It allows us to store and manipulate a collection of elements. In TypeScript, we can use the built-in Array class to achieve similar functionality.
To use ArrayList in TypeScript, we can create a class that extends the Array class and adds additional methods to it. For example, let’s create a class called ArrayList that extends the Array class and adds a method called add:
class ArrayList
add(element: T): void {
this.push(element);
}
}
In this example, we create a generic class called ArrayList that extends the Array class. We also add a method called add that takes an element of type T and pushes it to the end of the array.
Now, we can create an instance of the ArrayList class and use the add method to add elements to it:
const list = new ArrayList
list.add(1);
list.add(2);
list.add(3);
console.log(list); // [1, 2, 3]
In this example, we create an instance of the ArrayList class and add three numbers to it using the add method. We then log the contents of the array to the console.
In conclusion, we can use the built-in Array class in TypeScript to achieve similar functionality to Java ArrayList. By extending the Array class and adding additional methods, we can create our own ArrayList class in TypeScript.
Equivalent of Java ArrayList in TypeScript
In conclusion, TypeScript provides a powerful and efficient way to work with arrays through its built-in array type and various array methods. One of the most useful array methods in TypeScript is the equivalent of the Java ArrayList function, which allows developers to dynamically add or remove elements from an array. This method provides a flexible and convenient way to manipulate arrays in TypeScript, making it easier to write clean and concise code. Whether you are a seasoned Java developer or new to TypeScript, the equivalent ArrayList function is a valuable tool to have in your arsenal. So, if you’re looking to work with arrays in TypeScript, be sure to explore this powerful method and see how it can help you streamline your code and improve your productivity.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |