The Java HashMap function is a data structure that stores key-value pairs in a hash table. It allows for efficient retrieval and insertion of elements by using a hash function to map keys to their corresponding values. The HashMap class provides methods for adding, removing, and accessing elements, as well as iterating over the key-value pairs. It is commonly used in Java programming for tasks such as caching, indexing, and data lookup. Keep reading below to learn how to Java HashMap in Bash.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
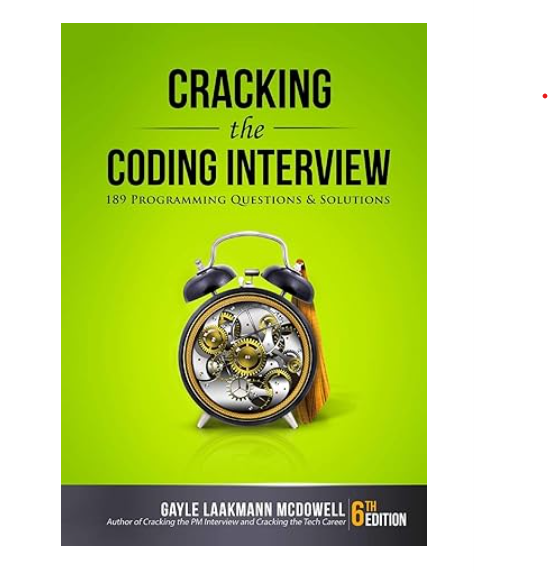
Java HashMap in Bash With Example Code
Java HashMap is a powerful data structure that allows you to store and retrieve key-value pairs efficiently. While it is a native data structure in Java, it is also possible to use it in Bash scripts with the help of the `jrunscript` command.
To use Java HashMap in Bash, you first need to create a Java code file that defines the HashMap and its methods. Here’s an example code:
import java.util.HashMap;
public class MyHashMap {
private HashMap
public MyHashMap() {
map = new HashMap
}
public void put(String key, String value) {
map.put(key, value);
}
public String get(String key) {
return map.get(key);
}
public boolean containsKey(String key) {
return map.containsKey(key);
}
public void remove(String key) {
map.remove(key);
}
}
Save this code in a file named `MyHashMap.java`.
Next, you need to compile this code into a class file using the `javac` command:
javac MyHashMap.java
This will create a `MyHashMap.class` file in the same directory.
Now, you can use this class in your Bash script using the `jrunscript` command:
#!/bin/bash
# Load the MyHashMap class
jrunscript -e 'load("MyHashMap.class")'
# Create a new instance of MyHashMap
jrunscript -e 'var map = new MyHashMap()'
# Add some key-value pairs
jrunscript -e 'map.put("key1", "value1")'
jrunscript -e 'map.put("key2", "value2")'
# Retrieve a value by key
jrunscript -e 'var value = map.get("key1")'
echo $value
# Check if a key exists
jrunscript -e 'var exists = map.containsKey("key2")'
echo $exists
# Remove a key-value pair
jrunscript -e 'map.remove("key1")'
This script creates a new instance of `MyHashMap`, adds some key-value pairs, retrieves a value by key, checks if a key exists, and removes a key-value pair.
Using Java HashMap in Bash can be a powerful tool for managing key-value data efficiently. With the `jrunscript` command, you can easily integrate Java code into your Bash scripts and take advantage of the many benefits of Java data structures.
Equivalent of Java HashMap in Bash
In conclusion, the equivalent Java HashMap function in Bash can be achieved through the use of associative arrays. Bash provides a simple and efficient way to store key-value pairs in memory, allowing for quick and easy access to data. By using the syntax “${array[key]}”, we can retrieve the value associated with a specific key in the array. Additionally, Bash provides a variety of built-in functions for manipulating associative arrays, such as “declare -A” for creating a new array and “unset” for removing a key-value pair. Overall, the use of associative arrays in Bash provides a powerful tool for managing data and can be a useful alternative to more complex data structures in other programming languages.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |