The Java HashMap function is a data structure that stores key-value pairs in a hash table. It allows for efficient retrieval and insertion of elements by using a hash function to map keys to their corresponding values. The HashMap class provides methods for adding, removing, and accessing elements, as well as iterating over the key-value pairs. It is commonly used in Java programming for tasks such as caching, indexing, and data lookup. Keep reading below to learn how to Java HashMap in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
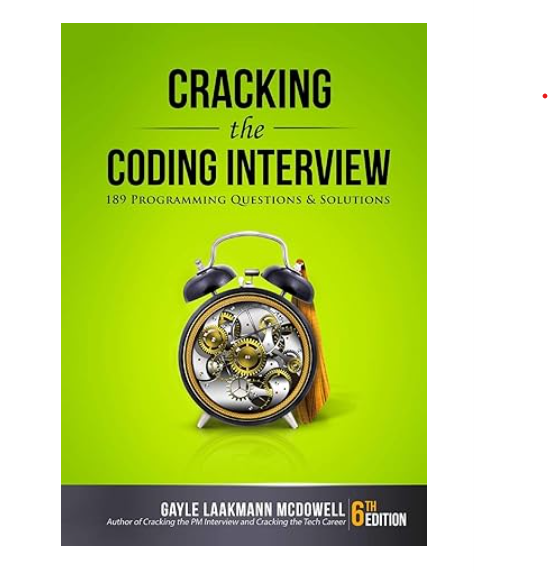
Java HashMap in C++ With Example Code
Java HashMap is a widely used data structure in Java programming language. It is used to store key-value pairs and provides constant time complexity for basic operations like insertion, deletion, and retrieval. However, C++ does not have a built-in HashMap data structure. In this blog post, we will discuss how to implement a HashMap in C++.
To implement a HashMap in C++, we can use an array of linked lists. Each element of the array will represent a bucket, and each bucket will contain a linked list of key-value pairs. The key-value pairs will be stored in nodes of the linked list.
To insert a key-value pair into the HashMap, we first calculate the hash value of the key. We then use this hash value to determine the bucket in which the key-value pair should be stored. We then traverse the linked list in the bucket to check if the key already exists. If it does, we update the value associated with the key. If it does not, we create a new node and add it to the linked list.
To retrieve a value from the HashMap, we first calculate the hash value of the key. We then use this hash value to determine the bucket in which the key-value pair should be stored. We then traverse the linked list in the bucket to find the node with the matching key. If we find the node, we return the value associated with the key. If we do not find the node, we return null.
Here is an example implementation of a HashMap in C++:
using namespace std; class HashMap { int hash(int key) { public: int get(int key) { int main() {
#include
#include
private:
static const int num_buckets = 10;
list
return key % num_buckets;
}
void put(int key, int value) {
int bucket = hash(key);
for (auto it = buckets[bucket].begin(); it != buckets[bucket].end(); it++) {
if (it->first == key) {
it->second = value;
return;
}
}
buckets[bucket].push_back(make_pair(key, value));
}
int bucket = hash(key);
for (auto it = buckets[bucket].begin(); it != buckets[bucket].end(); it++) {
if (it->first == key) {
return it->second;
}
}
return -1;
}
};
HashMap map;
map.put(1, 10);
map.put(2, 20);
map.put(3, 30);
cout << map.get(1) << endl; // Output: 10
cout << map.get(2) << endl; // Output: 20
cout << map.get(3) << endl; // Output: 30
cout << map.get(4) << endl; // Output: -1
return 0;
}
In this example, we have implemented a HashMap using an array of linked lists. We have defined a hash function that calculates the hash value of the key. We have also defined the put() and get() methods to insert and retrieve key-value pairs from the HashMap. Finally, we have tested the HashMap by inserting three key-value pairs and retrieving their values. We have also tested the get() method with a key that does not exist in the HashMap.
Equivalent of Java HashMap in C++
In conclusion, the C++ language provides a similar function to Java's HashMap through the use of the unordered_map container. This container allows for efficient storage and retrieval of key-value pairs, similar to the HashMap in Java. While the syntax and implementation may differ slightly between the two languages, the core functionality remains the same. As a programmer, it is important to understand the similarities and differences between different programming languages and their data structures in order to choose the best tool for the job. Whether you are working in Java or C++, the HashMap and unordered_map containers provide a powerful and flexible way to manage your data.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |