The Java HashMap function is a data structure that stores key-value pairs in a hash table. It allows for efficient retrieval and insertion of elements by using a hash function to map keys to their corresponding values. The HashMap class provides methods for adding, removing, and accessing elements, as well as iterating over the key-value pairs. It is commonly used in Java programming for tasks such as caching, indexing, and storing data. Keep reading below to learn how to Java HashMap in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
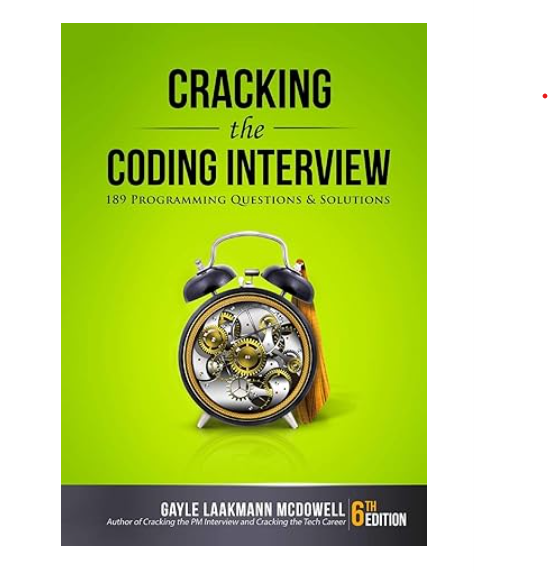
Java HashMap in Python With Example Code
Java HashMap is a popular data structure used in Java programming language. It is used to store key-value pairs and provides constant time complexity for basic operations like get and put. However, if you are working with Python, you might wonder how to implement Java HashMap in Python. In this blog post, we will explore how to do that.
Python provides a built-in data structure called dictionary which is similar to Java HashMap. It also stores key-value pairs and provides constant time complexity for basic operations. Therefore, we can use Python dictionary to implement Java HashMap in Python.
To create a Java HashMap in Python, we can simply create a dictionary and use it in a similar way as we use Java HashMap. For example, to create a Java HashMap with integer keys and string values, we can use the following code:
# create a Java HashMap in Python
java_map = {1: "one", 2: "two", 3: "three"}
# get value for a key
value = java_map.get(2)
print(value) # output: "two"
# put a new key-value pair
java_map[4] = "four"
print(java_map) # output: {1: "one", 2: "two", 3: "three", 4: "four"}
As you can see, we can use the get method to get the value for a key and the [] operator to put a new key-value pair. This is similar to how we use Java HashMap.
In conclusion, we can implement Java HashMap in Python using the built-in dictionary data structure. This allows us to use the same key-value pair data structure in both Java and Python programming languages.
Equivalent of Java HashMap in Python
In conclusion, Python’s equivalent to Java’s HashMap function is the dictionary data type. Both data structures allow for efficient key-value pair storage and retrieval, with the added benefit of dynamic resizing. While the syntax and implementation may differ slightly between the two languages, the core functionality remains the same. As a Python developer, understanding the dictionary data type and its various methods is crucial for efficient and effective programming. With this knowledge, you can confidently store and retrieve data in your Python programs with ease.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |