The Java String charAt function is a method that returns the character at a specified index position within a string. The index position starts at 0 for the first character in the string and increases by 1 for each subsequent character. The charAt function takes an integer argument that represents the index position of the desired character. If the index is out of range, the function throws an IndexOutOfBoundsException. The returned value is a single character represented as a char data type. This function is useful for accessing and manipulating individual characters within a string. Keep reading below to learn how to Java String charAt in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
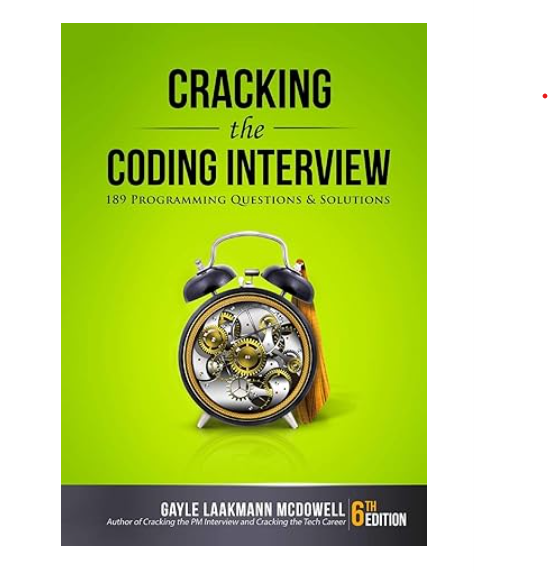
Java String charAt in C# With Example Code
Java’s String class has a method called charAt() that returns the character at a specified index in a string. C# does not have a built-in equivalent to this method, but it can be easily replicated using the string’s indexer property.
To use the indexer property, simply access the string as if it were an array and provide the index of the character you want to retrieve. For example:
string myString = "Hello, world!";
char myChar = myString[1]; // retrieves the character 'e'
In this example, the variable myChar will contain the character ‘e’, which is at index 1 in the string “Hello, world!”.
It’s important to note that attempting to access an index that is outside the bounds of the string will result in an IndexOutOfRangeException. To avoid this, you can check the length of the string before attempting to access an index:
string myString = "Hello, world!";
if (myString.Length > 1)
{
char myChar = myString[1]; // retrieves the character 'e'
}
In this example, the if statement checks if the length of the string is greater than 1 before attempting to retrieve the character at index 1. If the length is less than or equal to 1, the code inside the if statement will not be executed, preventing an IndexOutOfRangeException.
Using the string indexer property in C# is a simple and effective way to replicate the functionality of Java’s charAt() method.
Equivalent of Java String charAt in C#
In conclusion, the equivalent function of Java’s String charAt in C# is the String’s Indexer. Both functions serve the same purpose of returning the character at a specific index in a string. However, the syntax and implementation of the two functions differ slightly. While Java’s charAt function uses parentheses and an integer index to access a character, C#’s Indexer uses square brackets and an integer index to achieve the same result. Despite these differences, both functions are essential tools for manipulating strings in their respective programming languages. As a programmer, it is important to understand the similarities and differences between these functions to effectively use them in your code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |