The Java String charAt function is a method that returns the character at a specified index position within a string. The index position starts at 0 for the first character in the string and increases by 1 for each subsequent character. The charAt function takes an integer argument that represents the index position of the desired character. If the index is out of range, the function will throw an IndexOutOfBoundsException. The returned value is a single character, which can be stored in a char variable or used in other operations. This function is useful for accessing and manipulating individual characters within a string. Keep reading below to learn how to Java String charAt in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
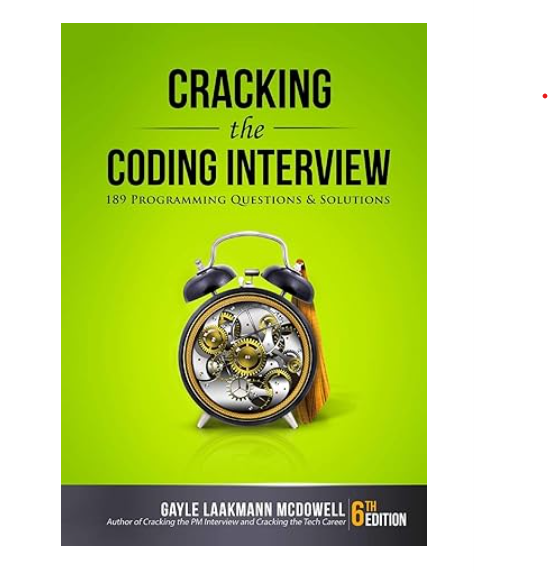
Java String charAt in Rust With Example Code
Java String charAt is a method that returns the character at a specified index in a string. Rust, being a different programming language, does not have the same method. However, there are ways to achieve the same functionality in Rust.
One way to get the character at a specific index in a Rust string is to use the `chars()` method. This method returns an iterator over the characters in the string. We can then use the `nth()` method to get the character at the specified index.
Here’s an example code snippet:
let my_string = "hello";
let my_char = my_string.chars().nth(1).unwrap();
println!("{}", my_char);
In this example, we have a string “hello”. We use the `chars()` method to get an iterator over the characters in the string. We then use the `nth()` method to get the character at index 1 (which is the second character in the string). Finally, we use `unwrap()` to get the actual character value.
Another way to achieve the same functionality is to use Rust’s array indexing syntax. Rust strings are essentially just arrays of characters, so we can use the indexing syntax to get the character at a specific index.
Here’s an example code snippet:
let my_string = "hello";
let my_char = my_string[1..2].to_string();
println!("{}", my_char);
In this example, we use the indexing syntax to get the character at index 1 (which is the second character in the string). We use the `to_string()` method to convert the character to a string so that we can print it.
In conclusion, while Rust does not have a `charAt()` method like Java, there are ways to achieve the same functionality using Rust’s built-in methods and syntax.
Equivalent of Java String charAt in Rust
In conclusion, the Rust programming language provides a similar function to Java’s String charAt function through its indexing syntax. By using square brackets and passing in the index of the desired character, Rust developers can easily access and manipulate individual characters within a string. While the syntax may differ slightly from Java, the functionality remains the same. This demonstrates the versatility and flexibility of Rust as a language, allowing developers to achieve similar results in different ways. Overall, the equivalent of Java’s String charAt function in Rust is a useful tool for string manipulation and is a testament to the power and adaptability of the Rust programming language.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |