The Java String charAt function is a method that returns the character at a specified index position within a string. The index position starts at 0 for the first character in the string and increases by 1 for each subsequent character. The charAt function takes an integer argument that represents the index position of the desired character. If the index is out of range, the function will throw an IndexOutOfBoundsException. The returned value is a single character, which can be stored in a char variable or used in other string operations. Keep reading below to learn how to Java String charAt in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
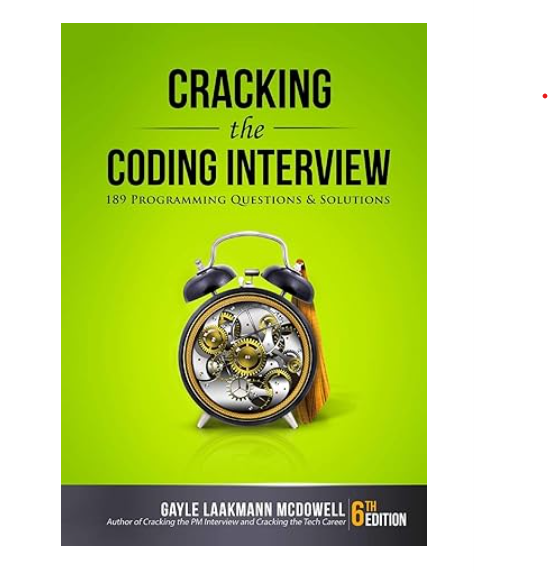
Java String charAt in TypeScript With Example Code
Java String charAt is a method that returns the character at a specified index in a string. TypeScript is a superset of JavaScript that adds optional static typing and other features to the language. In this blog post, we will discuss how to use Java String charAt in TypeScript.
To use Java String charAt in TypeScript, you first need to create a string variable. For example:
let str: string = "Hello World";
Once you have a string variable, you can use the charAt method to get the character at a specific index. The charAt method takes an index as a parameter and returns the character at that index. For example:
let char: string = str.charAt(0);
In this example, the char variable will contain the character “H” because it is the character at index 0 in the “Hello World” string.
It is important to note that the charAt method is zero-indexed, meaning that the first character in a string is at index 0, the second character is at index 1, and so on.
If you try to access an index that is outside the range of the string, the charAt method will return an empty string. For example:
let char: string = str.charAt(100);
In this example, the char variable will contain an empty string because there is no character at index 100 in the “Hello World” string.
In conclusion, Java String charAt is a useful method for getting the character at a specific index in a string. In TypeScript, you can use the charAt method by creating a string variable and calling the method with an index parameter. Just remember that the charAt method is zero-indexed and will return an empty string if you try to access an index outside the range of the string.
Equivalent of Java String charAt in TypeScript
In conclusion, the equivalent function of Java’s String charAt in TypeScript is the charAt() method. This method allows developers to access a specific character in a string by providing the index of the character. It is a useful tool for manipulating strings in TypeScript, and it can be used in a variety of applications. By understanding the charAt() method and how it works, developers can write more efficient and effective code in TypeScript. Whether you are a beginner or an experienced developer, the charAt() method is an essential tool to have in your toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |