The Java String compareTo function is used to compare two strings lexicographically. It returns an integer value that indicates the relationship between the two strings. If the first string is lexicographically greater than the second string, it returns a positive integer. If the first string is lexicographically smaller than the second string, it returns a negative integer. If both strings are equal, it returns 0. The comparison is based on the Unicode value of each character in the strings. The function is case-sensitive, meaning that uppercase letters are considered greater than lowercase letters. Keep reading below to learn how to Java String compareTo in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
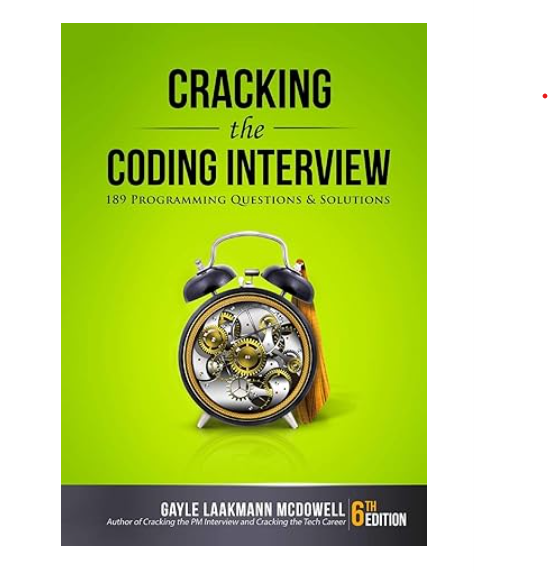
Java String compareTo in C# With Example Code
Java’s String class has a method called compareTo() that compares two strings lexicographically. This method returns an integer value that represents the difference between the two strings. In C#, there is no direct equivalent to the Java compareTo() method, but we can achieve the same functionality using the CompareTo() method of the String class.
The CompareTo() method compares the current string object to another string object and returns an integer value that indicates the relationship between the two strings. If the current string object is less than the other string object, the method returns a negative integer. If the current string object is greater than the other string object, the method returns a positive integer. If the two strings are equal, the method returns zero.
Here’s an example of how to use the CompareTo() method in C#:
string str1 = "hello";
string str2 = "world";
int result = str1.CompareTo(str2);
if (result < 0)
{
Console.WriteLine("str1 is less than str2");
}
else if (result > 0)
{
Console.WriteLine("str1 is greater than str2");
}
else
{
Console.WriteLine("str1 is equal to str2");
}
In this example, we create two string objects, “hello” and “world”, and compare them using the CompareTo() method. The result variable will contain a negative integer because “hello” is less than “world”. We then use an if-else statement to print out the relationship between the two strings.
In summary, while C# does not have a direct equivalent to Java’s String compareTo() method, we can achieve the same functionality using the CompareTo() method of the String class.
Equivalent of Java String compareTo in C#
In conclusion, the Java String compareTo function and its equivalent in C# are both powerful tools for comparing strings in their respective programming languages. While the syntax and implementation may differ slightly, the fundamental purpose and functionality remain the same. By understanding the similarities and differences between these two functions, developers can effectively utilize them to compare strings and improve the efficiency and accuracy of their code. Whether you are working with Java or C#, the compareTo function is an essential tool for any programmer looking to compare strings in their code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |