The Java String compareTo function is used to compare two strings lexicographically. It returns an integer value that indicates the relationship between the two strings. If the first string is lexicographically greater than the second string, it returns a positive integer. If the first string is lexicographically smaller than the second string, it returns a negative integer. If both strings are equal, it returns 0. The comparison is based on the Unicode value of each character in the strings. The function is case-sensitive, meaning that uppercase letters are considered greater than lowercase letters. Keep reading below to learn how to Java String compareTo in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
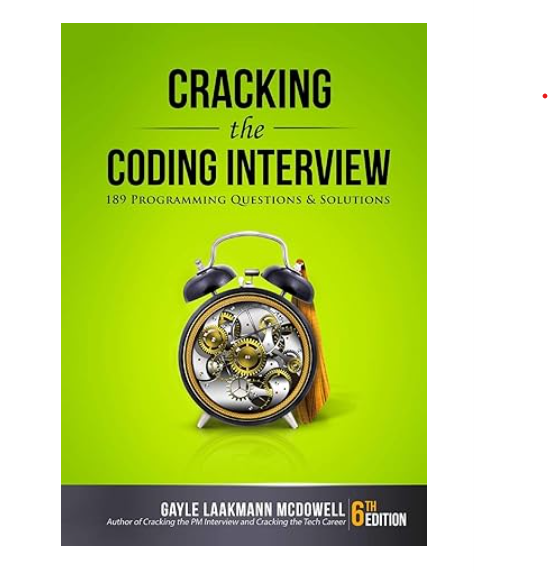
Java String compareTo in Go With Example Code
Java’s String class has a method called compareTo() that compares two strings lexicographically. This method returns an integer value that represents the difference between the two strings. In Go, we can achieve the same functionality using the strings.Compare() function.
The strings.Compare() function takes two string arguments and returns an integer value. If the first string is less than the second string, it returns a negative integer. If the first string is greater than the second string, it returns a positive integer. If the two strings are equal, it returns 0.
Here’s an example code snippet that demonstrates the usage of strings.Compare() function:
package main
import (
"fmt"
"strings"
)
func main() {
str1 := "hello"
str2 := "world"
result := strings.Compare(str1, str2)
if result < 0 {
fmt.Printf("%s is less than %s\n", str1, str2)
} else if result > 0 {
fmt.Printf("%s is greater than %s\n", str1, str2)
} else {
fmt.Printf("%s and %s are equal\n", str1, str2)
}
}
In this example, we have two strings “hello” and “world”. We use the strings.Compare() function to compare these two strings and store the result in the variable “result”. We then use an if-else statement to print the appropriate message based on the result of the comparison.
By using the strings.Compare() function, we can achieve the same functionality as Java’s String compareTo() method in Go.
Equivalent of Java String compareTo in Go
In conclusion, the equivalent function of Java’s String compareTo in Go is the strings.Compare() function. This function compares two strings lexicographically and returns an integer value that indicates whether the first string is less than, equal to, or greater than the second string. It is a simple and efficient way to compare strings in Go, and it can be used in a variety of applications, from sorting to searching. By understanding the similarities and differences between these two functions, developers can easily transition between Java and Go and create robust and efficient applications. Overall, the strings.Compare() function is a valuable tool for any Go developer looking to compare strings in their code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |