The Java String compareTo function is used to compare two strings lexicographically. It returns an integer value that indicates the relationship between the two strings. If the first string is lexicographically greater than the second string, it returns a positive integer. If the first string is lexicographically smaller than the second string, it returns a negative integer. If both strings are equal, it returns 0. The comparison is based on the Unicode value of each character in the strings. The function is case-sensitive, meaning that uppercase letters are considered greater than lowercase letters. Keep reading below to learn how to Java String compareTo in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
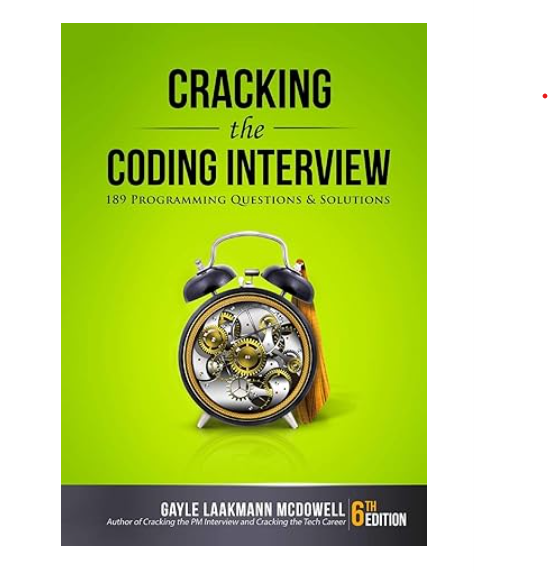
Java String compareTo in Javascript With Example Code
Java’s String class has a method called compareTo() that compares two strings lexicographically. This method returns an integer value that represents the difference between the two strings. In JavaScript, there is no built-in method for comparing strings lexicographically like Java’s compareTo() method. However, we can write our own function to achieve the same result.
To compare two strings lexicographically in JavaScript, we can use the localeCompare() method. This method compares two strings and returns a number that indicates whether the first string comes before, after, or is equal to the second string in the sort order.
Here’s an example of how to use the localeCompare() method to compare two strings:
const str1 = "apple";
const str2 = "banana";
const result = str1.localeCompare(str2);
if (result < 0) {
console.log("str1 comes before str2");
} else if (result > 0) {
console.log("str2 comes before str1");
} else {
console.log("str1 and str2 are equal");
}
In this example, we first declare two variables, str1 and str2, and assign them the values “apple” and “banana”, respectively. We then use the localeCompare() method to compare the two strings and store the result in the variable result.
We then use an if-else statement to check the value of result. If result is less than 0, it means that str1 comes before str2 in the sort order. If result is greater than 0, it means that str2 comes before str1 in the sort order. If result is equal to 0, it means that str1 and str2 are equal.
In conclusion, while JavaScript doesn’t have a built-in method for comparing strings lexicographically like Java’s compareTo() method, we can use the localeCompare() method to achieve the same result.
Equivalent of Java String compareTo in Javascript
In conclusion, the equivalent function of Java’s String compareTo in JavaScript is the localeCompare() method. This method compares two strings and returns a value indicating whether one string is less than, equal to, or greater than the other string based on the language-specific sorting order. It also takes into account the locale and any special characters or accents in the strings. By using the localeCompare() method, developers can easily compare strings in JavaScript with the same functionality as the compareTo() method in Java. Overall, understanding the similarities and differences between these two methods can help developers write more efficient and effective code in both languages.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |