The Java String compareTo function is used to compare two strings lexicographically. It returns an integer value that indicates the relationship between the two strings. If the first string is lexicographically greater than the second string, it returns a positive integer. If the first string is lexicographically smaller than the second string, it returns a negative integer. If both strings are equal, it returns 0. The comparison is based on the Unicode value of each character in the strings. The function is case-sensitive, meaning that uppercase letters are considered greater than lowercase letters. Keep reading below to learn how to Java String compareTo in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
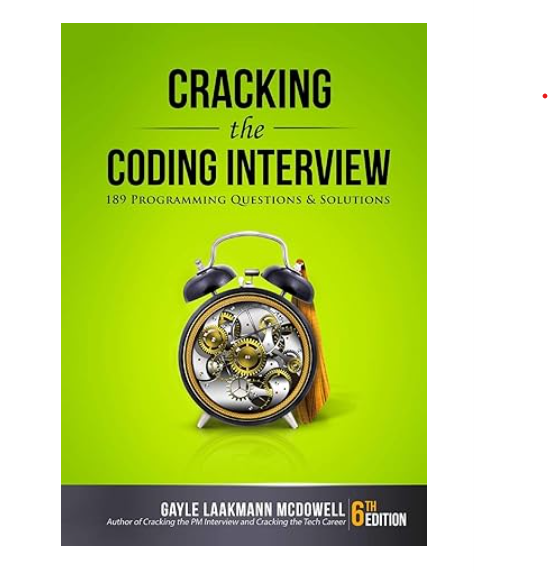
Java String compareTo in Python With Example Code
Java and Python are two of the most popular programming languages in the world. While they have many similarities, there are also some differences between them. One of these differences is the way they handle strings. In Java, there is a method called compareTo() that is used to compare two strings. In Python, there is no direct equivalent to this method, but there are ways to achieve the same result.
To compare two strings in Java using the compareTo() method, you simply call the method on one string and pass in the other string as a parameter. The method returns an integer value that indicates the relationship between the two strings. If the two strings are equal, the method returns 0. If the first string is lexicographically less than the second string, the method returns a negative integer. If the first string is lexicographically greater than the second string, the method returns a positive integer.
In Python, you can achieve the same result using the built-in function ord(). This function returns the Unicode code point of a character. You can use this function to compare two strings by iterating over each character in the strings and comparing their Unicode code points. If the code points are equal, move on to the next character. If the code point of the first string is less than the code point of the second string, return a negative integer. If the code point of the first string is greater than the code point of the second string, return a positive integer. If you reach the end of one of the strings and all the characters are equal, return 0.
Here is an example of how to compare two strings in Python using the ord() function:
def compare_strings(str1, str2):
i = 0
while i < len(str1) and i < len(str2):
if ord(str1[i]) < ord(str2[i]):
return -1
elif ord(str1[i]) > ord(str2[i]):
return 1
i += 1
if len(str1) < len(str2):
return -1
elif len(str1) > len(str2):
return 1
else:
return 0
In conclusion, while Java and Python handle strings differently, there are ways to achieve the same result. By using the compareTo() method in Java and the ord() function in Python, you can compare two strings and determine their relationship to each other.
Equivalent of Java String compareTo in Python
In conclusion, the equivalent function of Java’s String compareTo in Python is the built-in function called “cmp”. Both functions compare two strings and return an integer value indicating their relative order. However, it is important to note that the cmp function is deprecated in Python 3 and is no longer available. Instead, the recommended approach is to use the comparison operators such as “<", ">“, and “==”. These operators can be used to compare strings in Python and return a boolean value indicating the result of the comparison. Overall, understanding the similarities and differences between these functions can help developers write more efficient and effective code in both Java and Python.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |