The Java String compareToIgnoreCase function is used to compare two strings lexicographically, ignoring case differences. It returns an integer value that indicates the relationship between the two strings. If the two strings are equal, it returns 0. If the first string is lexicographically greater than the second string, it returns a positive integer. If the first string is lexicographically less than the second string, it returns a negative integer. This function is useful when comparing strings in a case-insensitive manner, such as when sorting or searching for strings in a collection. Keep reading below to learn how to Java String compareToIgnoreCase in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
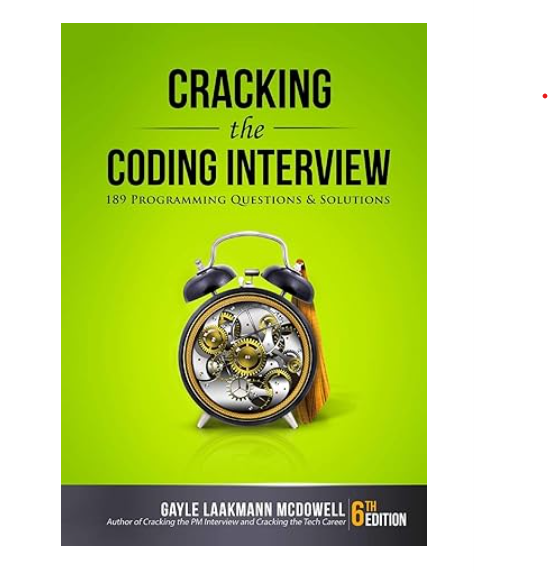
Java String compareToIgnoreCase in C# With Example Code
Java’s String class has a method called `compareToIgnoreCase()` that compares two strings lexicographically, ignoring case differences. This method is useful when you want to compare two strings without considering their case. In C#, there is no built-in method that does exactly the same thing, but you can easily create your own.
To create a method that compares two strings ignoring case, you can use the `String.Compare()` method and pass in the `StringComparison.OrdinalIgnoreCase` parameter. Here’s an example:
public static bool CompareStringsIgnoreCase(string str1, string str2)
{
return String.Compare(str1, str2, StringComparison.OrdinalIgnoreCase) == 0;
}
In this example, the `CompareStringsIgnoreCase()` method takes two string parameters and returns a boolean value indicating whether the two strings are equal, ignoring case.
To use this method, you can simply call it and pass in the two strings you want to compare:
string str1 = "Hello";
string str2 = "hello";
bool isEqual = CompareStringsIgnoreCase(str1, str2);
Console.WriteLine(isEqual); // Output: True
In this example, the `isEqual` variable will be set to `true` because the two strings are equal, ignoring case.
In summary, while C# doesn’t have a built-in method for comparing strings ignoring case like Java’s `compareToIgnoreCase()`, you can easily create your own method using `String.Compare()` and the `StringComparison.OrdinalIgnoreCase` parameter.
Equivalent of Java String compareToIgnoreCase in C#
In conclusion, the equivalent function of Java’s String compareToIgnoreCase in C# is the String.Compare method with the StringComparison.OrdinalIgnoreCase parameter. This method compares two strings and returns an integer value that indicates their relative position in the sort order. The StringComparison.OrdinalIgnoreCase parameter ensures that the comparison is case-insensitive, just like the compareToIgnoreCase function in Java. It is important to note that while the syntax and implementation may differ between Java and C#, the functionality remains the same. As a developer, it is essential to understand the similarities and differences between programming languages to effectively translate code from one language to another. With this knowledge, you can confidently use the String.Compare method in C# to achieve the same results as the compareToIgnoreCase function in Java.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |