The Java String concat function is used to concatenate two or more strings together. It takes one or more string arguments and returns a new string that is the concatenation of all the input strings. The concat function can be called on any string object and can be used to join strings together in a variety of ways. It is a simple and efficient way to combine strings in Java programming. Keep reading below to learn how to Java String concat in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
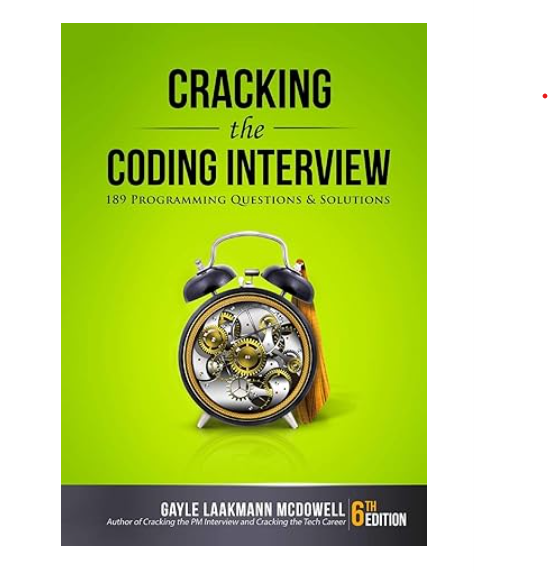
Java String concat in Javascript With Example Code
Java String Concat in JavaScript
If you’re familiar with Java, you may know about the String.concat() method. This method is used to concatenate two strings together. In JavaScript, there is no built-in method for concatenating strings in the same way. However, there are a few ways to achieve the same result.
One way to concatenate strings in JavaScript is to use the + operator. This operator can be used to concatenate two or more strings together. For example:
var str1 = "Hello";
var str2 = "World";
var result = str1 + " " + str2;
console.log(result); // Output: "Hello World"
In this example, we declare two variables, str1 and str2, and assign them the values “Hello” and “World”, respectively. We then use the + operator to concatenate the two strings together, with a space in between. The result is stored in the variable result, and then output to the console.
Another way to concatenate strings in JavaScript is to use the concat() method. This method is similar to the Java String.concat() method. For example:
var str1 = "Hello";
var str2 = "World";
var result = str1.concat(" ", str2);
console.log(result); // Output: "Hello World"
In this example, we declare two variables, str1 and str2, and assign them the values “Hello” and “World”, respectively. We then use the concat() method to concatenate the two strings together, with a space in between. The result is stored in the variable result, and then output to the console.
In conclusion, there are a few ways to concatenate strings in JavaScript, including using the + operator and the concat() method. Both methods achieve the same result as the Java String.concat() method.
Equivalent of Java String concat in Javascript
In conclusion, the equivalent function of Java’s String concat() in JavaScript is the concatenation operator (+). Both functions serve the same purpose of combining two or more strings into a single string. However, the syntax and usage of the two functions differ slightly. While Java’s concat() function requires the string to be concatenated as an argument, the JavaScript concatenation operator allows for the direct concatenation of strings without the need for an argument. It is important to note that the concatenation operator in JavaScript can also be used to concatenate strings with other data types such as numbers and booleans. Overall, understanding the equivalent function of Java’s String concat() in JavaScript is essential for developers who work with both languages.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |