The Java String concat function is used to concatenate two or more strings together. It takes one or more string arguments and returns a new string that is the concatenation of all the input strings. The original strings are not modified, and the new string is created as a separate object in memory. The concat function can be called using the dot notation on a string object, or it can be called as a static method of the String class. It is a commonly used function in Java programming for combining strings in various applications. Keep reading below to learn how to Java String concat in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
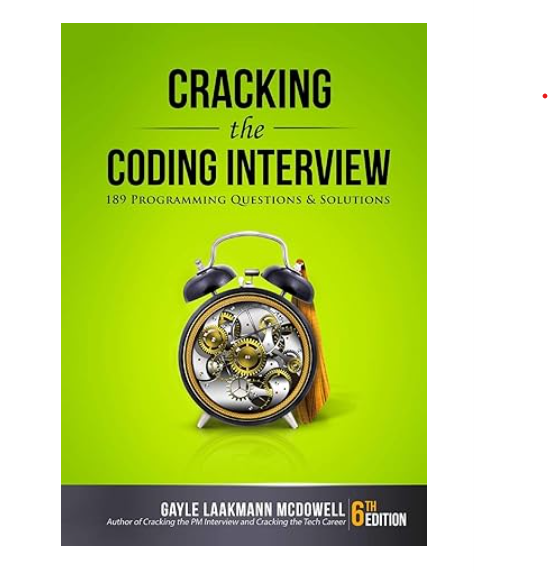
Java String concat in Rust With Example Code
Java developers who are transitioning to Rust may find themselves wondering how to perform string concatenation in Rust. In Java, string concatenation is typically done using the `+` operator or the `concat()` method. Rust, on the other hand, has a different syntax for string concatenation.
In Rust, you can concatenate strings using the `+` operator or the `format!()` macro. The `+` operator works by taking two string slices and concatenating them into a new `String` object. Here’s an example:
let hello = "Hello, ";
let world = "world!";
let hello_world = hello + world;
println!("{}", hello_world);
This will output “Hello, world!” to the console.
If you need to concatenate more than two strings, you can chain multiple `+` operators together. For example:
let hello = "Hello, ";
let name = "John";
let exclamation = "!";
let greeting = hello + name + exclamation;
println!("{}", greeting);
This will output “Hello, John!” to the console.
Alternatively, you can use the `format!()` macro to concatenate strings. This macro works by taking a format string and a list of arguments, and returning a new `String` object. Here’s an example:
let hello = "Hello, ";
let name = "John";
let exclamation = "!";
let greeting = format!("{}{}{}", hello, name, exclamation);
println!("{}", greeting);
This will output “Hello, John!” to the console.
In summary, Rust provides two main ways to concatenate strings: using the `+` operator or the `format!()` macro. Both methods are easy to use and provide flexibility for concatenating multiple strings.
Equivalent of Java String concat in Rust
In conclusion, Rust provides a powerful and efficient way to concatenate strings using the `format!` macro. This macro allows for easy string interpolation and formatting, making it a great alternative to Java’s `String.concat()` function. Additionally, Rust’s ownership and borrowing system ensures that string concatenation is done in a safe and efficient manner, without any unnecessary memory allocations. Overall, Rust’s approach to string concatenation is a great example of the language’s focus on performance, safety, and ease of use. Whether you’re a seasoned Rust developer or just getting started, the `format!` macro is a valuable tool to have in your arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |