The Java String contains function is a method that is used to check whether a particular sequence of characters is present in a given string or not. It returns a boolean value of true if the specified sequence of characters is found in the string, and false otherwise. The method takes a single argument, which is the sequence of characters to be searched for in the string. It is case-sensitive, meaning that it will only match the exact sequence of characters provided. The contains function is commonly used in string manipulation and searching operations in Java programming. Keep reading below to learn how to Java String contains in Bash.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
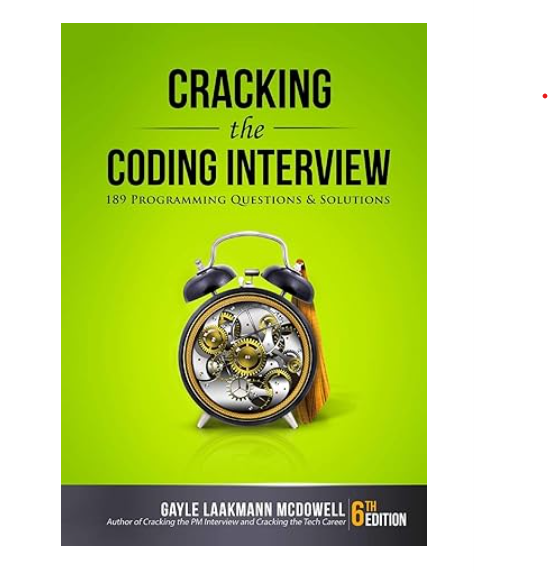
Java String contains in Bash With Example Code
Java String contains is a useful method for checking if a string contains a specific substring. However, what if you need to use this functionality in a Bash script? In this blog post, we will explore how to use Java String contains in Bash.
First, we need to understand that Bash does not have a built-in method for checking if a string contains a substring. However, we can use the Java command-line tool to achieve this functionality.
To use Java String contains in Bash, we need to create a Java program that takes two arguments: the string we want to search in and the substring we want to search for. Here is an example Java program:
public class StringContains {
public static void main(String[] args) {
String str = args[0];
String substr = args[1];
if (str.contains(substr)) {
System.out.println("true");
} else {
System.out.println("false");
}
}
}
We can compile this program using the following command:
javac StringContains.java
Now, we can use this program in our Bash script. Here is an example Bash script that uses the Java String contains method:
#!/bin/bash
str="Hello, world!"
substr="world"
contains=$(java StringContains "$str" "$substr")
if [ "$contains" = "true" ]; then
echo "The string contains the substring."
else
echo "The string does not contain the substring."
fi
In this script, we set the variables str and substr to the string we want to search in and the substring we want to search for, respectively. We then use the Java StringContains program to check if str contains substr. The output of the program is stored in the contains variable.
Finally, we use an if statement to check if contains is equal to “true”. If it is, we print a message saying that the string contains the substring. Otherwise, we print a message saying that the string does not contain the substring.
In conclusion, while Bash does not have a built-in method for checking if a string contains a substring, we can use the Java command-line tool to achieve this functionality. By creating a Java program that takes two arguments, we can use the Java String contains method in our Bash scripts.
Equivalent of Java String contains in Bash
In conclusion, the Bash shell provides a powerful set of tools for working with strings. While there is no direct equivalent to the Java String contains function in Bash, we can use various techniques to achieve the same result. We can use the grep command with regular expressions, the test command with pattern matching, or the [[ operator with wildcard patterns. Each of these methods has its own advantages and disadvantages, and the choice depends on the specific use case. By understanding these techniques, we can write more efficient and effective Bash scripts that manipulate strings with ease.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |