The Java String contains function is a method that is used to check whether a particular sequence of characters is present in a given string or not. It returns a boolean value of true if the specified sequence of characters is found in the string, and false otherwise. The method takes a single argument, which is the sequence of characters to be searched for in the string. It is case-sensitive, meaning that it will only match the exact sequence of characters provided. The contains function is commonly used in string manipulation and searching operations in Java programming. Keep reading below to learn how to Java String contains in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
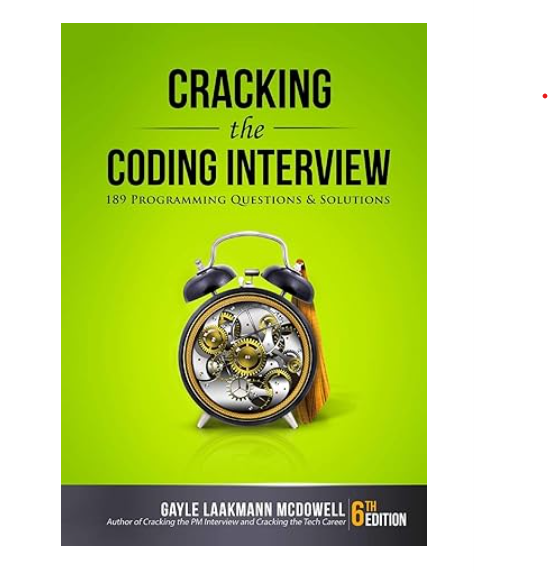
Java String contains in C++ With Example Code
Java String contains in C++
In Java, the `String` class has a method called `contains()` that checks whether a string contains a specified sequence of characters. However, C++ does not have a built-in method for this. In this blog post, we will explore different ways to achieve the same functionality in C++.
Method 1: Using the find() function
One way to check if a string contains a substring in C++ is to use the `find()` function. This function returns the position of the first occurrence of the substring in the string. If the substring is not found, it returns `string::npos`. Here’s an example:
#include
#include
int main() {
std::string str = "Hello, world!";
std::string substr = "world";
if (str.find(substr) != std::string::npos) {
std::cout << "Substring found!" << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
In this example, we create a string `str` and a substring `substr`. We then use the `find()` function to check if `str` contains `substr`. If it does, we print "Substring found!". Otherwise, we print "Substring not found.".
Method 2: Using the strstr() function
Another way to check if a string contains a substring in C++ is to use the `strstr()` function. This function is part of the C standard library and is used to find the first occurrence of a substring in a string. Here's an example:
#include
#include
int main() {
char str[] = "Hello, world!";
char substr[] = "world";
if (strstr(str, substr) != nullptr) {
std::cout << "Substring found!" << std::endl;
} else {
std::cout << "Substring not found." << std::endl;
}
return 0;
}
In this example, we create two character arrays `str` and `substr`. We then use the `strstr()` function to check if `str` contains `substr`. If it does, we print "Substring found!". Otherwise, we print "Substring not found.".
Conclusion
In this blog post, we explored two different ways to check if a string contains a substring in C++. The `find()` function is part of the C++ standard library and is recommended for use with `std::string` objects. The `strstr()` function is part of the C standard library and is recommended for use with character arrays.
Equivalent of Java String contains in C++
In conclusion, the equivalent function to Java's String contains() in C++ is the find() function. While the syntax and usage may differ slightly, both functions serve the same purpose of searching for a specific substring within a larger string. It is important to note that the find() function returns the index of the first occurrence of the substring, or a value of string::npos if the substring is not found. By understanding the similarities and differences between these two functions, developers can effectively utilize them in their code to achieve the desired results.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |