The Java String contentEquals() function is used to compare the content of two strings. It returns a boolean value indicating whether the content of the two strings is equal or not. This function takes a CharSequence object as an argument and compares it with the content of the string. If the content of the CharSequence object is equal to the content of the string, then it returns true, otherwise, it returns false. This function is useful when we need to compare the content of two strings without considering their case or any other differences. Keep reading below to learn how to Java String contentEquals in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
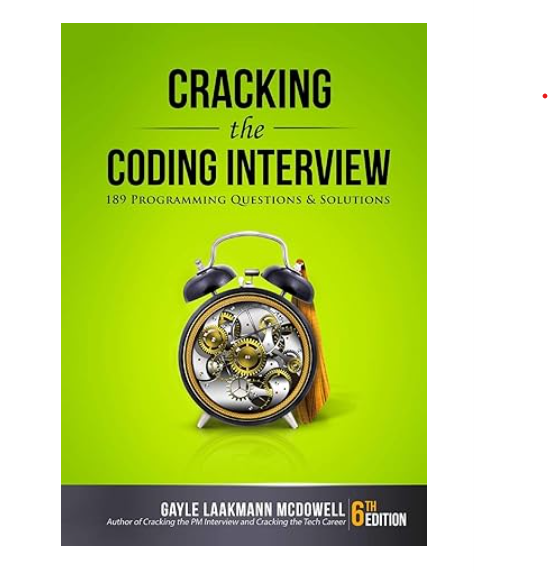
Java String contentEquals in C# With Example Code
Java’s String class has a method called `contentEquals` that allows you to compare the contents of two strings. This method is not available in C#, but there are ways to achieve the same functionality.
One way to compare the contents of two strings in C# is to use the `Equals` method. This method compares the values of two strings and returns a boolean value indicating whether they are equal or not. Here’s an example:
string str1 = "Hello";
string str2 = "World";
bool isEqual = str1.Equals(str2);
In this example, the `Equals` method is used to compare the values of `str1` and `str2`. The `isEqual` variable will be set to `false` because the two strings have different values.
Another way to compare the contents of two strings in C# is to use the `CompareTo` method. This method compares the values of two strings and returns an integer value indicating their relative order. If the two strings are equal, the method returns `0`. Here’s an example:
string str1 = "Hello";
string str2 = "hello";
int result = str1.CompareTo(str2);
In this example, the `CompareTo` method is used to compare the values of `str1` and `str2`. The `result` variable will be set to a value greater than `0` because the two strings have different values.
In summary, while C# does not have a `contentEquals` method like Java’s String class, you can achieve the same functionality using the `Equals` and `CompareTo` methods.
Equivalent of Java String contentEquals in C#
In conclusion, the Java String contentEquals function and its equivalent in C# provide developers with a powerful tool for comparing the contents of two strings. While the syntax and implementation may differ slightly between the two languages, the core functionality remains the same. By using these functions, developers can ensure that their code is robust and reliable, and that their applications are able to handle a wide range of input data. Whether you are working in Java or C#, understanding how to use these functions effectively is an essential skill for any developer.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |