The Java String contentEquals() function is used to compare the content of two strings. It returns a boolean value indicating whether the content of the two strings is equal or not. This function takes a CharSequence object as an argument and compares it with the content of the string. If the content of the CharSequence object is equal to the content of the string, then it returns true, otherwise, it returns false. This function is useful when we need to compare the content of two strings without considering their case or any other differences. Keep reading below to learn how to Java String contentEquals in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
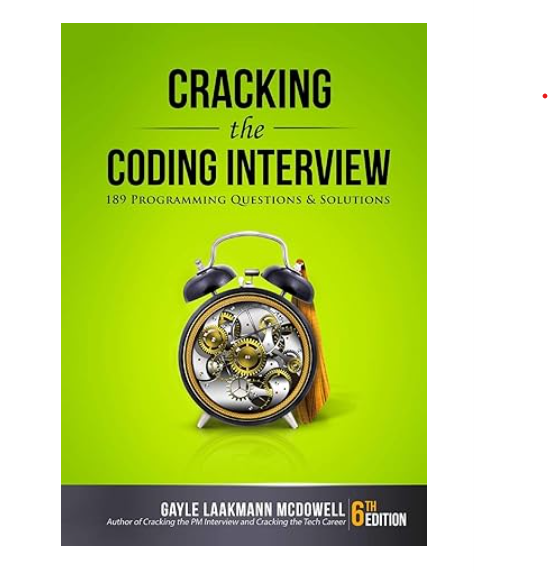
Java String contentEquals in Go With Example Code
Java’s `String` class provides a method called `contentEquals` that allows you to compare the contents of two strings. If you’re working with Go and need to perform a similar comparison, you can use the `bytes.Equal` function.
Here’s an example of how to use `bytes.Equal` to compare two strings in Go:
import "bytes"
func main() {
str1 := "hello"
str2 := "world"
if bytes.Equal([]byte(str1), []byte(str2)) {
fmt.Println("The strings are equal")
} else {
fmt.Println("The strings are not equal")
}
}
In this example, we’re using the `bytes.Equal` function to compare the contents of `str1` and `str2`. We first convert the strings to byte slices using the `[]byte` conversion, and then pass them to `bytes.Equal`.
If the strings are equal, the program will output “The strings are equal”. Otherwise, it will output “The strings are not equal”.
Keep in mind that `bytes.Equal` performs a byte-by-byte comparison of the two slices. If you need to perform a case-insensitive comparison or ignore leading/trailing whitespace, you’ll need to implement that logic yourself.
Equivalent of Java String contentEquals in Go
In conclusion, the equivalent function of Java’s String contentEquals in Go is the EqualFold method. Both functions are used to compare the content of two strings without considering their case sensitivity. The EqualFold method is a built-in function in Go’s standard library, making it easily accessible for developers. It is important to note that while the two functions serve the same purpose, they have different syntax and usage. Therefore, it is essential for developers to understand the differences between the two and use them appropriately in their code. Overall, the EqualFold method is a useful tool for Go developers who need to compare strings without considering case sensitivity.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |