The Java String contentEquals() function is used to compare the content of two strings. It returns a boolean value indicating whether the content of the two strings is equal or not. This function takes a CharSequence object as an argument and compares it with the content of the string. If the content of the CharSequence object is equal to the content of the string, then it returns true, otherwise, it returns false. This function is useful when we need to compare the content of two strings without considering their case or any other differences. Keep reading below to learn how to Java String contentEquals in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
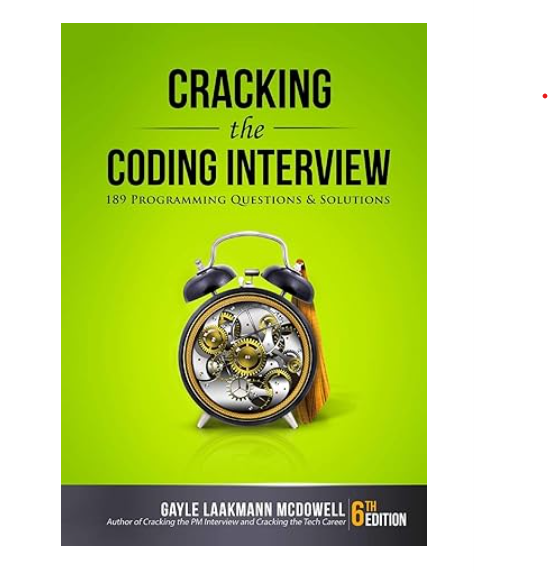
Java String contentEquals in Javascript With Example Code
Java’s String class has a method called `contentEquals` that compares the contents of two strings. This method can be useful in certain situations, such as when you need to compare two strings for equality but don’t want to use the `==` operator.
In JavaScript, there is no built-in method that directly corresponds to `contentEquals`. However, you can achieve the same functionality by using the `localeCompare` method.
The `localeCompare` method compares two strings and returns a number indicating whether the first string is less than, equal to, or greater than the second string. If the two strings are equal, `localeCompare` returns 0.
To use `localeCompare` to compare the contents of two strings in JavaScript, you can do the following:
const str1 = "hello";
const str2 = "hello";
if (str1.localeCompare(str2) === 0) {
console.log("The strings are equal");
} else {
console.log("The strings are not equal");
}
In this example, `str1` and `str2` are both set to “hello”. The `localeCompare` method is used to compare the two strings, and the result is checked to see if it is equal to 0. If it is, the strings are considered equal and a message is logged to the console. If it is not, a different message is logged.
By using `localeCompare` in this way, you can achieve the same functionality as Java’s `contentEquals` method in JavaScript.
Equivalent of Java String contentEquals in Javascript
In conclusion, the equivalent function of Java’s String contentEquals in JavaScript is the localeCompare method. Both functions compare the content of two strings and return a boolean value indicating whether they are equal or not. However, the localeCompare method in JavaScript provides additional functionality by allowing you to specify the locale and compare strings based on language-specific rules. This can be particularly useful when working with multilingual applications. Overall, understanding the similarities and differences between these two functions can help you write more efficient and effective code in both Java and JavaScript.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |