The Java String endsWith function is a method that is used to check whether a given string ends with a specified suffix or not. It returns a boolean value of true if the string ends with the specified suffix, and false otherwise. The endsWith function takes a single argument, which is the suffix that needs to be checked. This function is case-sensitive, which means that it considers the case of the characters in the string and the suffix. It is commonly used in string manipulation and validation tasks, such as checking file extensions or validating email addresses. Keep reading below to learn how to Java String endsWith in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
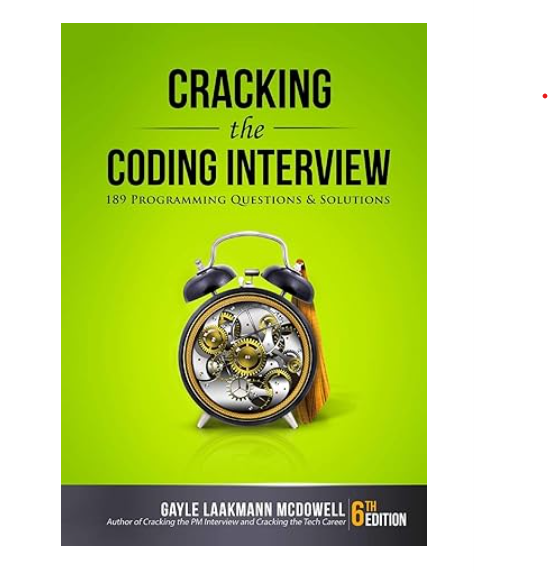
Java String endsWith in C# With Example Code
Java’s `String` class has a method called `endsWith()` that checks whether a given string ends with a specified suffix. This method is very useful when you need to perform operations on strings based on their endings. In C#, there is no direct equivalent of the `endsWith()` method, but you can achieve the same functionality using the `Substring()` method.
To check whether a string ends with a specified suffix in C#, you can use the following code:
string str = "Hello, world!";
string suffix = "world!";
if (str.Substring(str.Length - suffix.Length) == suffix)
{
Console.WriteLine("The string ends with the suffix.");
}
else
{
Console.WriteLine("The string does not end with the suffix.");
}
In this code, we first define a string `str` and a suffix `suffix`. We then use the `Substring()` method to extract the last `suffix.Length` characters from `str`. We compare this substring to the `suffix` string using the `==` operator to check whether `str` ends with `suffix`.
If the comparison is true, we print a message indicating that the string ends with the suffix. Otherwise, we print a message indicating that the string does not end with the suffix.
Using this approach, you can easily check whether a string ends with a specified suffix in C#.
Equivalent of Java String endsWith in C#
In conclusion, the equivalent function of Java’s endsWith() in C# is the String.EndsWith() method. This method is used to determine whether a string ends with a specified suffix. It takes a string parameter that represents the suffix to search for and returns a boolean value indicating whether the string ends with the specified suffix. The String.EndsWith() method is a useful tool for C# developers who need to perform string operations. It provides a simple and efficient way to check whether a string ends with a particular substring. By using this method, developers can easily manipulate strings and perform various operations on them. Overall, the String.EndsWith() method is a valuable addition to the C# language and is a great alternative to Java’s endsWith() function. It is easy to use, efficient, and provides accurate results, making it an essential tool for any C# developer.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |