The Java String endsWith function is a method that is used to check whether a given string ends with a specified suffix or not. It returns a boolean value of true if the string ends with the specified suffix, and false otherwise. The endsWith function takes a single argument, which is the suffix that needs to be checked. This function is case-sensitive, which means that it considers the case of the characters in the string and the suffix. It is commonly used in string manipulation and validation tasks, such as checking file extensions or validating email addresses. Keep reading below to learn how to Java String endsWith in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
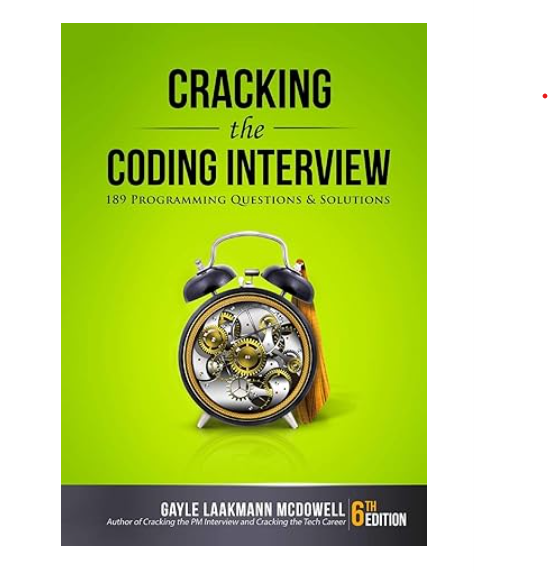
Java String endsWith in C++ With Example Code
Java’s String class has a method called `endsWith()` that checks whether a string ends with a specified suffix. This method is very useful when you need to perform operations on the end of a string. In C++, there is no built-in method for this, but we can easily create our own.
To create a function that checks whether a string ends with a specified suffix, we can use the `substr()` method to extract the end of the string and compare it to the suffix. Here’s an example implementation:
bool endsWith(std::string const& str, std::string const& suffix) {
if (suffix.size() > str.size()) return false;
return std::equal(suffix.rbegin(), suffix.rend(), str.rbegin());
}
In this implementation, we first check if the suffix is longer than the string. If it is, we know that the string cannot end with the suffix, so we return false. Otherwise, we use the `std::equal()` algorithm to compare the suffix to the end of the string. We use `rbegin()` and `rend()` to iterate over the string and suffix in reverse order, so that we can compare the end of the string to the suffix.
To use this function, simply call it with the string and suffix you want to check:
std::string str = "hello world";
std::string suffix = "world";
if (endsWith(str, suffix)) {
std::cout << "The string ends with the suffix" << std::endl;
}
This will output "The string ends with the suffix", since the string "hello world" does indeed end with the suffix "world".
With this implementation, you can easily check whether a string ends with a specified suffix in C++, just like you would with Java's `endsWith()` method.
Equivalent of Java String endsWith in C++
In conclusion, the equivalent function to Java's String endsWith() in C++ is the std::string::substr() function. By using this function, we can extract a substring from the end of a string and compare it to another string to determine if it ends with that substring. While the syntax may be slightly different from Java, the functionality is the same and can be easily implemented in C++ programs. By understanding the similarities and differences between these two programming languages, we can become more versatile and effective programmers.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |