The Java String endsWith function is a method that is used to check whether a given string ends with a specified suffix or not. It returns a boolean value of true if the string ends with the specified suffix, and false otherwise. The endsWith function takes a single argument, which is the suffix that needs to be checked. This function is case-sensitive, which means that it considers the case of the characters in the string and the suffix. The endsWith function is commonly used in string manipulation and validation tasks, such as checking file extensions or validating email addresses. Keep reading below to learn how to Java String endsWith in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
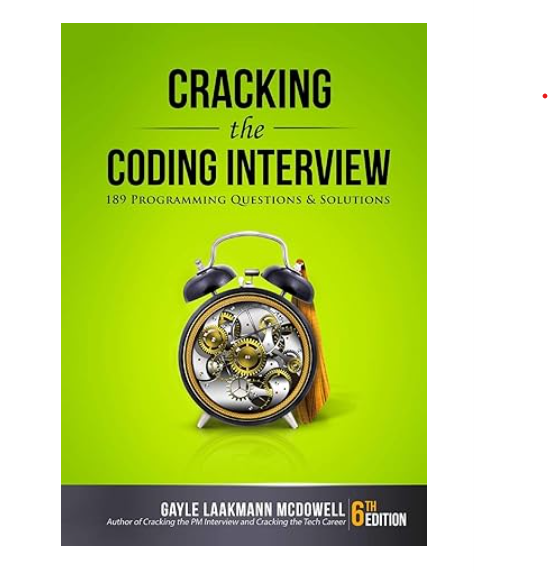
Java String endsWith in Go With Example Code
Java’s `endsWith` method is a useful tool for checking if a string ends with a specific suffix. However, if you’re working in Go, you may be wondering how to achieve the same functionality. Fortunately, Go provides a simple solution with the `strings` package.
To check if a string ends with a specific suffix in Go, you can use the `strings.HasSuffix` function. This function takes two arguments: the string you want to check, and the suffix you want to check for. It returns a boolean value indicating whether or not the string ends with the specified suffix.
Here’s an example of how to use `strings.HasSuffix` in Go:
package main
import (
"fmt"
"strings"
)
func main() {
str := "hello world"
suffix := "world"
if strings.HasSuffix(str, suffix) {
fmt.Println("String ends with suffix")
} else {
fmt.Println("String does not end with suffix")
}
}
In this example, we’re checking if the string “hello world” ends with the suffix “world”. If it does, we print “String ends with suffix”. If it doesn’t, we print “String does not end with suffix”.
Using `strings.HasSuffix` is a simple and effective way to check if a string ends with a specific suffix in Go.
Equivalent of Java String endsWith in Go
In conclusion, the Go programming language provides a simple and efficient way to check if a string ends with a specific substring using the `strings.HasSuffix()` function. This function works similarly to the Java `endsWith()` function and takes two arguments: the string to check and the substring to search for. By using this function, Go developers can easily determine if a string ends with a specific substring without having to write complex code. Overall, the `strings.HasSuffix()` function is a valuable tool for any Go developer who needs to work with strings and wants to ensure their code is efficient and easy to read.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |