The Java String endsWith function is a method that is used to check whether a given string ends with a specified suffix or not. It returns a boolean value of true if the string ends with the specified suffix, and false otherwise. The endsWith function takes a single argument, which is the suffix that needs to be checked. This function is case-sensitive, which means that it considers the case of the characters in the string and the suffix. It is commonly used in string manipulation and validation tasks, such as checking file extensions or validating email addresses. Keep reading below to learn how to Java String endsWith in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
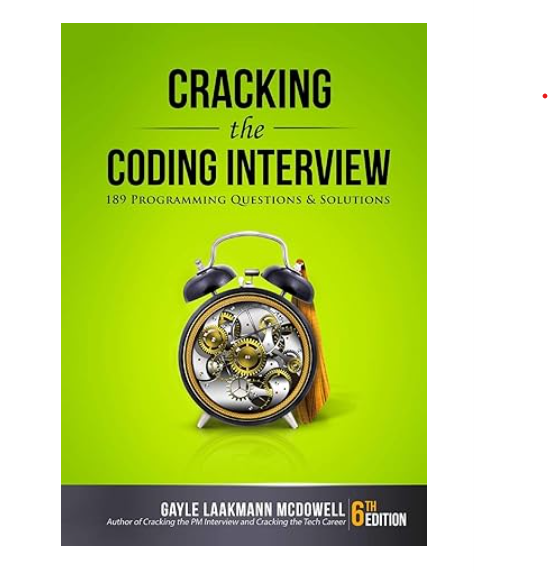
Java String endsWith in Kotlin With Example Code
Java String’s `endsWith()` method is a useful tool for checking if a string ends with a specific suffix. In Kotlin, this method can be used in a similar way.
To use `endsWith()` in Kotlin, you can simply call the method on a string and pass in the suffix you want to check for. The method will return a boolean value indicating whether or not the string ends with the specified suffix.
Here’s an example of how to use `endsWith()` in Kotlin:
val str = "Hello, world!"
val suffix = "ld!"
if (str.endsWith(suffix)) {
println("The string ends with $suffix")
} else {
println("The string does not end with $suffix")
}
In this example, we create a string `str` and a suffix `suffix`. We then use the `endsWith()` method to check if `str` ends with `suffix`. If it does, we print a message indicating that the string ends with the suffix. If it doesn’t, we print a message indicating that the string does not end with the suffix.
Overall, `endsWith()` is a simple and useful method for checking if a string ends with a specific suffix in Kotlin.
Equivalent of Java String endsWith in Kotlin
In conclusion, the Kotlin programming language provides a convenient and efficient way to check if a string ends with a specific character or sequence of characters. The equivalent function to Java’s endsWith() is the endsWith() function in Kotlin’s String class. This function takes a string parameter and returns a boolean value indicating whether the original string ends with the specified string. By using this function, Kotlin developers can easily and reliably check the end of a string without having to write complex code. Overall, the endsWith() function is a valuable tool for Kotlin developers looking to streamline their string manipulation tasks.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |