The Java String endsWith function is a method that is used to check whether a given string ends with a specified suffix or not. It returns a boolean value of true if the string ends with the specified suffix, and false otherwise. The endsWith function takes a single argument, which is the suffix that needs to be checked. This function is case-sensitive, which means that it considers the case of the characters in the string and the suffix. It is commonly used in string manipulation and validation tasks, such as checking file extensions or validating email addresses. Keep reading below to learn how to Java String endsWith in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
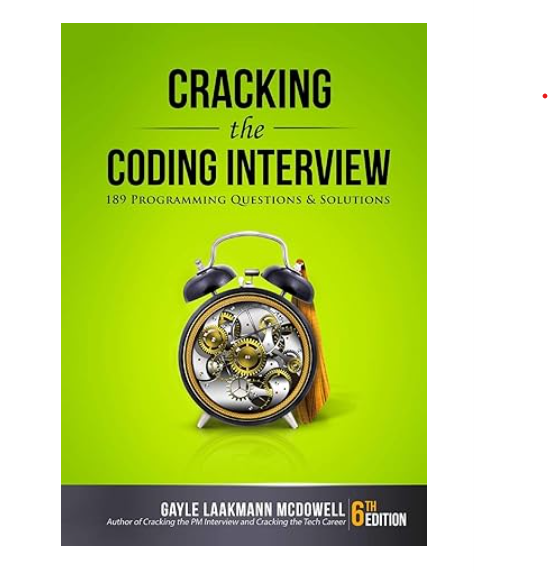
Java String endsWith in Rust With Example Code
Java’s `endsWith()` method is a useful tool for checking if a string ends with a specific suffix. If you’re working in Rust and need to perform a similar operation, you might be wondering how to achieve the same functionality. Fortunately, Rust provides a simple solution for this problem.
To check if a string ends with a specific suffix in Rust, you can use the `ends_with()` method. This method takes a string slice as an argument and returns a boolean value indicating whether the original string ends with the provided suffix.
Here’s an example of how to use `ends_with()` in Rust:
let my_string = "hello world";
let suffix = "world";
if my_string.ends_with(suffix) {
println!("The string ends with {}", suffix);
} else {
println!("The string does not end with {}", suffix);
}
In this example, we create a string called `my_string` and a suffix called `suffix`. We then use the `ends_with()` method to check if `my_string` ends with `suffix`. If it does, we print a message indicating that the string ends with the suffix. If it doesn’t, we print a message indicating that the string does not end with the suffix.
Overall, using `ends_with()` in Rust is a straightforward way to check if a string ends with a specific suffix. By using this method, you can easily perform this common operation in your Rust code.
Equivalent of Java String endsWith in Rust
In conclusion, Rust provides a powerful and efficient way to check if a string ends with a specific substring using the `ends_with` function. This function is similar to the `endsWith` function in Java and allows developers to easily check if a string ends with a specific pattern without having to write complex code. With Rust’s focus on performance and safety, the `ends_with` function is optimized to provide fast and reliable results. Whether you are a seasoned Rust developer or just getting started, the `ends_with` function is a valuable tool to have in your programming arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |