The Java String equals function is a method used to compare two strings and determine if they are equal. It returns a boolean value of true if the two strings are identical in terms of their characters and false if they are not. The comparison is case-sensitive, meaning that uppercase and lowercase letters are considered different. The equals function is commonly used in Java programming to check if two strings are equal before performing certain operations or making decisions based on their values. Keep reading below to learn how to Java String equals in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
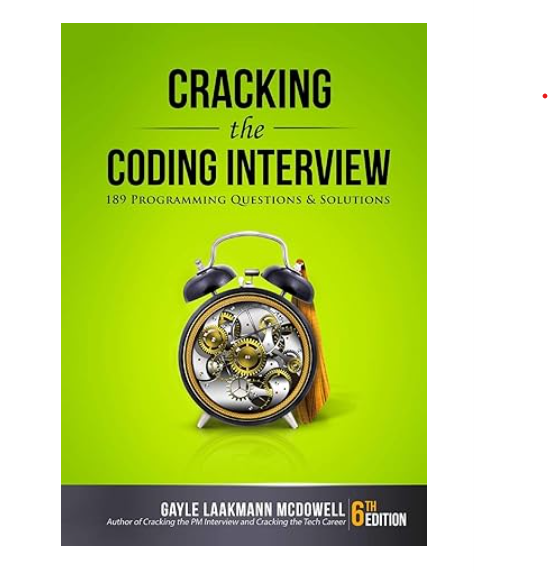
Java String equals in C++ With Example Code
Java’s String class has a method called “equals” that is used to compare two strings for equality. In C++, there is no built-in method for comparing strings in the same way. However, there are several ways to achieve the same result.
One way to compare two strings in C++ is to use the “compare” method of the string class. This method returns an integer value that indicates whether the two strings are equal, greater than, or less than each other. Here is an example:
#include
#include
using namespace std;
int main() {
string str1 = "Hello";
string str2 = "World";
if (str1.compare(str2) == 0) {
cout << "The two strings are equal." << endl;
} else {
cout << "The two strings are not equal." << endl;
}
return 0;
}
Another way to compare two strings in C++ is to use the "==" operator. This operator can be used to compare two strings directly, without the need for a separate method. Here is an example:
#include
#include
using namespace std;
int main() {
string str1 = "Hello";
string str2 = "World";
if (str1 == str2) {
cout << "The two strings are equal." << endl;
} else {
cout << "The two strings are not equal." << endl;
}
return 0;
}
In both of these examples, the output will be "The two strings are not equal." because the two strings are different.
Equivalent of Java String equals in C++
In conclusion, the equivalent function to Java's String equals() in C++ is the strcmp() function. While the syntax and usage may differ between the two languages, the underlying functionality remains the same. Both functions compare two strings and return a boolean value indicating whether they are equal or not. It is important to note that in C++, the strcmp() function returns 0 if the strings are equal, whereas in Java, the equals() function returns true. Understanding the similarities and differences between these functions can help developers write efficient and effective code in both languages.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |