The Java String equals function is a method used to compare two strings and determine if they are equal. It returns a boolean value of true if the two strings are identical in terms of their characters and false if they are not. The comparison is case-sensitive, meaning that uppercase and lowercase letters are considered different. The equals function is commonly used in Java programming to check if two strings are equal before performing certain operations or making decisions based on their values. Keep reading below to learn how to Java String equals in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
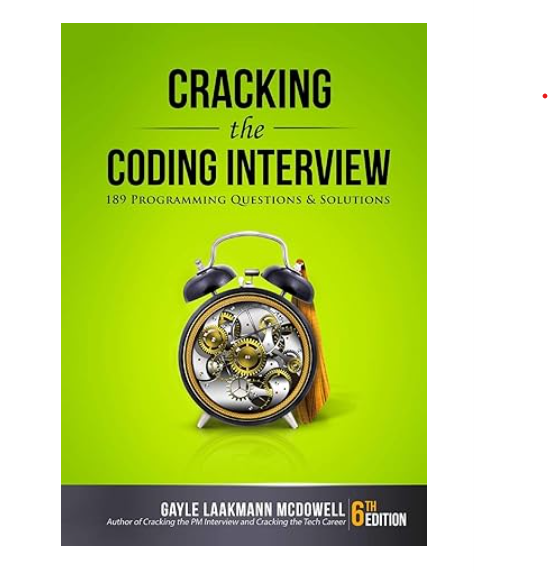
Java String equals in Go With Example Code
Java developers who are transitioning to Go may find themselves wondering how to perform a simple string comparison in Go. In Java, the `equals()` method is used to compare two strings for equality. In Go, the equivalent method is `==`.
To compare two strings in Go, simply use the `==` operator. Here’s an example:
package main
import "fmt"
func main() {
str1 := "hello"
str2 := "world"
if str1 == str2 {
fmt.Println("Strings are equal")
} else {
fmt.Println("Strings are not equal")
}
}
In this example, we declare two string variables `str1` and `str2`. We then use the `==` operator to compare the two strings. If the strings are equal, we print “Strings are equal”. Otherwise, we print “Strings are not equal”.
It’s important to note that in Go, strings are immutable. This means that once a string is created, it cannot be modified. If you need to modify a string, you’ll need to create a new string with the desired modifications.
In summary, comparing strings in Go is as simple as using the `==` operator. While Java developers may be used to using the `equals()` method, the `==` operator is the equivalent in Go.
Equivalent of Java String equals in Go
In conclusion, the equivalent function to Java’s String equals in Go is the == operator. While the syntax may be different, the functionality remains the same. Both functions compare two strings and return a boolean value indicating whether they are equal or not. It is important to note that in Go, the == operator can also be used to compare other data types, such as integers and floats. However, when comparing strings, it is recommended to use the == operator instead of other comparison operators, such as < or >, as they may not provide the expected results. Overall, understanding the equivalent function in Go is essential for developers who are transitioning from Java to Go or working with both languages simultaneously.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |