The Java String equals function is a method used to compare two strings and determine if they are equal. It returns a boolean value of true if the two strings are identical in terms of their characters and false if they are not. The comparison is case-sensitive, meaning that uppercase and lowercase letters are considered different. The equals function is commonly used in Java programming to check if two strings are equal before performing certain operations or making decisions based on their values. Keep reading below to learn how to Java String equals in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
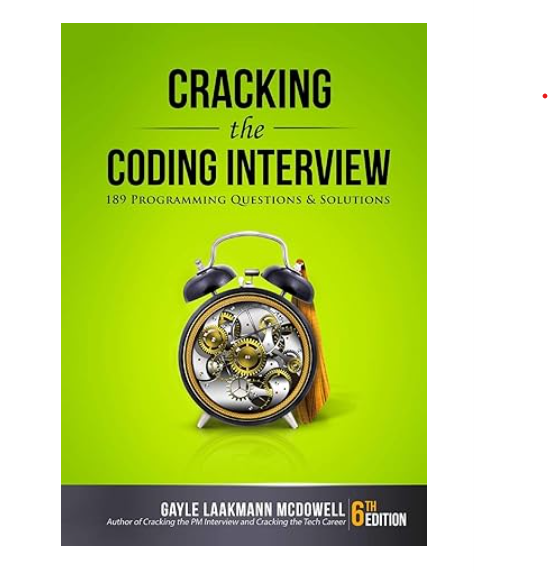
Java String equals in Kotlin With Example Code
Java String equals() method is used to compare two strings for equality. In Kotlin, the same method can be used to compare two strings. However, there are some differences in the syntax and usage of the method in Kotlin.
To compare two strings in Kotlin, you can use the equals() method of the String class. This method returns true if the two strings are equal, and false otherwise. Here is an example:
val str1 = "Hello"
val str2 = "World"
val str3 = "Hello"
if (str1.equals(str2)) {
println("str1 and str2 are equal")
} else {
println("str1 and str2 are not equal")
}
if (str1.equals(str3)) {
println("str1 and str3 are equal")
} else {
println("str1 and str3 are not equal")
}
In this example, we have three strings: str1, str2, and str3. We are using the equals() method to compare these strings. The first comparison is between str1 and str2, which are not equal. Therefore, the output will be “str1 and str2 are not equal”. The second comparison is between str1 and str3, which are equal. Therefore, the output will be “str1 and str3 are equal”.
It is important to note that the equals() method is case-sensitive. This means that “Hello” and “hello” are not equal. If you want to perform a case-insensitive comparison, you can use the equalsIgnoreCase() method instead.
In conclusion, the Java String equals() method can be used in Kotlin to compare two strings for equality. The syntax and usage of the method are the same in Kotlin as in Java.
Equivalent of Java String equals in Kotlin
In conclusion, the Kotlin programming language provides a simplified and more concise way of comparing strings using the `==` operator. This operator is equivalent to the Java `equals()` function and can be used to compare two strings for equality. Additionally, Kotlin also provides the `===` operator which can be used to compare two string references for equality. With these two operators, Kotlin developers can easily compare strings without having to worry about the nuances of the Java `equals()` function. Overall, Kotlin’s string comparison operators make it easier for developers to write clean and efficient code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |