The Java String equals function is a method used to compare two strings and determine if they are equal. It returns a boolean value of true if the two strings are identical in terms of their characters and false if they are not. The comparison is case-sensitive, meaning that uppercase and lowercase letters are considered different. The equals function is commonly used in Java programming to check if two strings are equal before performing certain operations or making decisions based on their values. Keep reading below to learn how to Java String equals in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
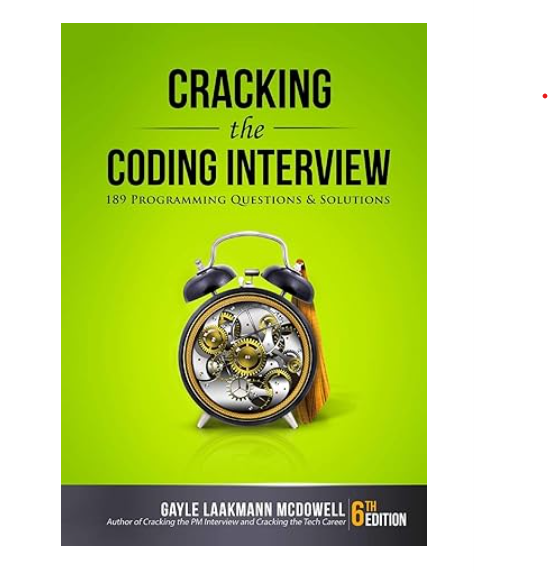
Java String equals in Rust With Example Code
Java and Rust are two popular programming languages with different syntax and features. If you are familiar with Java and want to learn how to compare strings in Rust, this post is for you.
In Java, you can compare two strings using the `equals()` method. This method compares the content of two strings and returns `true` if they are equal, and `false` otherwise. Here is an example:
String str1 = "hello";
String str2 = "world";
if (str1.equals(str2)) {
System.out.println("Strings are equal");
} else {
System.out.println("Strings are not equal");
}
In Rust, you can compare two strings using the `==` operator. This operator compares the content of two strings and returns `true` if they are equal, and `false` otherwise. Here is an example:
let str1 = "hello";
let str2 = "world";
if str1 == str2 {
println!("Strings are equal");
} else {
println!("Strings are not equal");
}
As you can see, the syntax for comparing strings in Rust is different from Java. However, the logic behind it is the same. You compare the content of two strings to determine if they are equal.
In conclusion, comparing strings in Rust is different from Java, but the logic behind it is the same. You can use the `==` operator in Rust to compare two strings, just like you use the `equals()` method in Java.
Equivalent of Java String equals in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to compare strings using the `==` operator. This operator works similarly to the Java `equals()` function, allowing developers to easily compare two strings for equality. Additionally, Rust’s `==` operator is designed to work with a wide range of string types, including both owned and borrowed strings. This makes it a versatile tool for any Rust developer working with strings. Overall, the `==` operator in Rust is a great alternative to the Java `equals()` function, providing a fast and reliable way to compare strings in your Rust code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |