The Java String equals function is a method used to compare two strings and determine if they are equal. It returns a boolean value of true if the two strings are identical in terms of their characters and false if they are not. The comparison is case-sensitive, meaning that uppercase and lowercase letters are considered different. The equals function is commonly used in Java programming to check if two strings are equal before performing certain operations or making decisions based on their values. Keep reading below to learn how to Java String equals in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
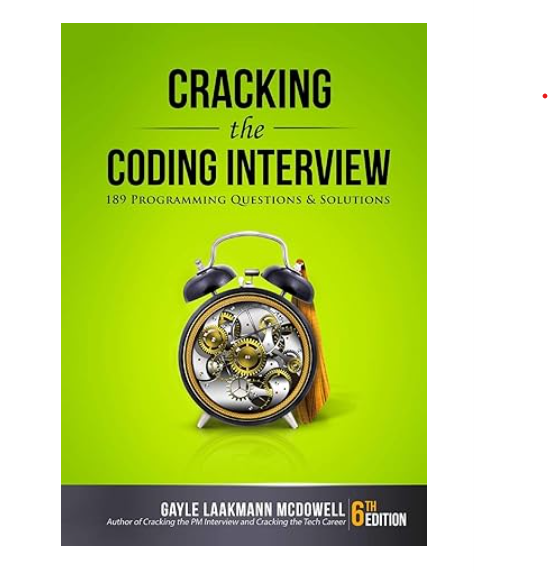
Java String equals in TypeScript With Example Code
Java’s String class has a method called “equals” that is used to compare two strings for equality. In TypeScript, there is no built-in method for comparing strings in the same way. However, we can create a function that performs the same task.
To create a function that compares two strings for equality in TypeScript, we can use the following code:
function stringEquals(str1: string, str2: string): boolean {
return str1 === str2;
}
This function takes two string parameters and returns a boolean value indicating whether the two strings are equal. It uses the strict equality operator (===) to compare the strings, which checks both the value and the type of the operands.
To use this function, simply call it with two string arguments:
const string1 = "hello";
const string2 = "world";
const string3 = "hello";
console.log(stringEquals(string1, string2)); // false
console.log(stringEquals(string1, string3)); // true
In this example, we create three string variables and compare them using the stringEquals function. The first comparison returns false because the two strings are not equal, while the second comparison returns true because the two strings have the same value.
By using this function, we can perform string comparisons in TypeScript that are equivalent to Java’s String equals method.
Equivalent of Java String equals in TypeScript
In conclusion, the equivalent function of Java’s String equals() in TypeScript is the strict equality operator (===). This operator compares two values for equality without performing any type conversion. It returns true if the values are equal and false otherwise. It is important to note that the strict equality operator only compares the values of the variables and not their types. Therefore, it is recommended to use the strict equality operator when comparing strings in TypeScript to ensure accurate results. By using this operator, developers can write more efficient and reliable code that will help them avoid common errors and bugs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |