The Java String equalsIgnoreCase function is a method that compares two strings while ignoring their case sensitivity. It returns a boolean value of true if the two strings are equal, regardless of whether they are in uppercase or lowercase. This function is useful when comparing user input or when comparing strings that may have been entered in different cases. It is important to note that this function only compares the content of the strings and not their memory location. Keep reading below to learn how to Java String equalsIgnoreCase in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
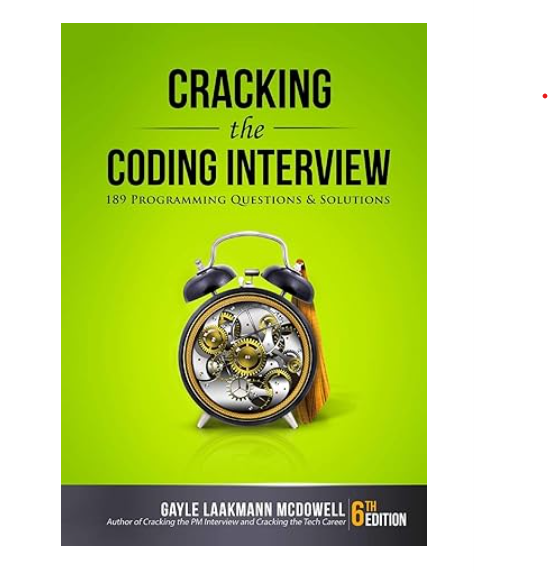
Java String equalsIgnoreCase in Javascript With Example Code
Java’s String class has a method called `equalsIgnoreCase()` that compares two strings while ignoring their case. This can be useful when you want to compare strings without worrying about whether they are in uppercase or lowercase.
In JavaScript, there is no built-in method for `equalsIgnoreCase()`, but you can easily create your own function to achieve the same result.
Here’s an example of how you can create a function that compares two strings while ignoring their case:
function equalsIgnoreCase(str1, str2) {
return str1.toLowerCase() === str2.toLowerCase();
}
In this function, we use the `toLowerCase()` method to convert both strings to lowercase before comparing them. This ensures that the comparison is case-insensitive.
You can use this function in your JavaScript code to compare strings without worrying about their case.
For example:
var str1 = "Hello";
var str2 = "hello";
if (equalsIgnoreCase(str1, str2)) {
console.log("The strings are equal (ignoring case)");
} else {
console.log("The strings are not equal (ignoring case)");
}
This will output “The strings are equal (ignoring case)” because the two strings are equal when their case is ignored.
In summary, while JavaScript doesn’t have a built-in `equalsIgnoreCase()` method like Java’s String class, you can easily create your own function to achieve the same result by converting both strings to lowercase before comparing them.
Equivalent of Java String equalsIgnoreCase in Javascript
In conclusion, the equivalent function of Java’s String equalsIgnoreCase in JavaScript is the toLowerCase() method. This method converts the string to lowercase and then compares it with another string, ignoring the case sensitivity. It is a useful function when you need to compare strings without worrying about the case of the characters. By using this method, you can simplify your code and make it more efficient. So, if you are working with JavaScript and need to compare strings without considering the case, the toLowerCase() method is the perfect solution for you.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |