The Java String format function is a method that allows you to create formatted strings by replacing placeholders with values. It takes a format string as its first argument, which contains placeholders for the values you want to insert. The placeholders are denoted by the percent sign followed by a letter that indicates the type of value to be inserted. For example, %s is used for strings, %d for integers, and %f for floating-point numbers. The method then takes additional arguments that correspond to the placeholders in the format string. These arguments are inserted into the string in the order they appear. The resulting string is returned by the method. Keep reading below to learn how to Java String format in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
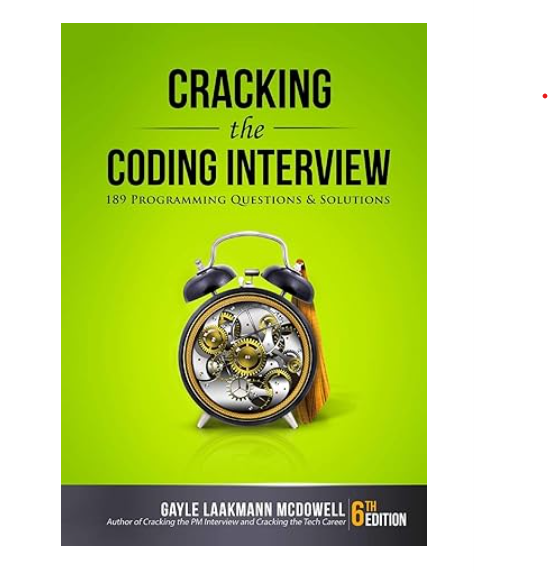
Java String format in C++ With Example Code
Java String format is a powerful tool that allows you to format strings in a variety of ways. If you’re working in C++, you might be wondering how to achieve the same functionality. Fortunately, there are several ways to format strings in C++ that are similar to Java’s String format.
One way to format strings in C++ is to use the sprintf function. This function works similarly to Java’s String format, allowing you to specify placeholders in a string and then replace them with values. Here’s an example:
char buffer[100];
int value = 42;
sprintf(buffer, "The answer is %d", value);
In this example, we’re using sprintf to format a string with a placeholder (%d) for an integer value. We then pass the value to sprintf as an argument, and it replaces the placeholder with the value.
Another way to format strings in C++ is to use the stringstream class. This class allows you to build a string by appending values to it, similar to how you might concatenate strings in Java. Here’s an example:
#include
#include
std::stringstream ss;
int value = 42;
ss << "The answer is " << value;
std::string result = ss.str();
In this example, we're using a stringstream object to build a string by appending a string literal and an integer value. We then convert the stringstream object to a string using the str() method.
Both of these methods provide similar functionality to Java's String format, allowing you to format strings with placeholders and replace them with values. By using these techniques, you can achieve the same level of flexibility and control over your string formatting in C++ as you would in Java.
Equivalent of Java String format in C++
In conclusion, the equivalent function to Java's String format in C++ is the sprintf function. This function allows for the formatting of strings with placeholders for variables, similar to Java's String format. However, it is important to note that the syntax and usage of sprintf may differ from Java's String format. It is also important to ensure that the placeholders and variables used in the sprintf function are of the correct data type to avoid errors. Overall, the sprintf function is a useful tool for formatting strings in C++ and can be a valuable addition to any programmer's toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |