The Java String format function is a method that allows you to create formatted strings by replacing placeholders with values. It takes a format string as its first argument, which contains placeholders for the values you want to insert. The placeholders are denoted by the percent sign followed by a letter that indicates the type of value to be inserted. For example, %s is used for strings, %d for integers, and %f for floating-point numbers. The method then takes additional arguments that correspond to the placeholders in the format string. These arguments are inserted into the string in the order they appear. The resulting string is returned by the method. Keep reading below to learn how to Java String format in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
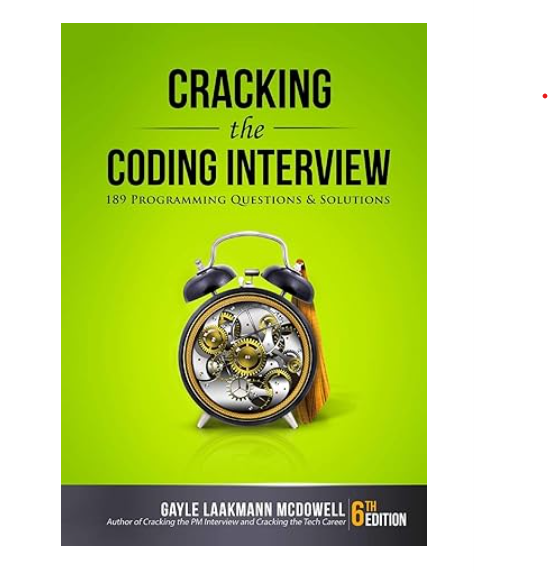
Java String format in Go With Example Code
Java developers who are transitioning to Go may find themselves wondering how to format strings in Go. Fortunately, Go has a similar syntax to Java for string formatting.
To format a string in Go, you can use the `fmt.Sprintf()` function. This function works similarly to Java’s `String.format()` method.
Here’s an example of how to use `fmt.Sprintf()` to format a string in Go:
name := "John"
age := 30
formattedString := fmt.Sprintf("My name is %s and I am %d years old.", name, age)
fmt.Println(formattedString)
In this example, we’re using `fmt.Sprintf()` to create a formatted string that includes the `name` and `age` variables.
The `%s` and `%d` placeholders in the string indicate where the values of `name` and `age` should be inserted. The order of the placeholders corresponds to the order of the variables passed to `fmt.Sprintf()`.
You can also use other formatting verbs in Go, such as `%f` for floating-point numbers and `%t` for booleans. You can find a full list of formatting verbs in the Go documentation.
Overall, formatting strings in Go is similar to formatting strings in Java. By using `fmt.Sprintf()`, you can easily create formatted strings that include variables and other values.
Equivalent of Java String format in Go
In conclusion, the equivalent Java String format function in Go is the fmt.Sprintf() function. This function allows developers to format strings in a similar way to the Java String format function, using placeholders and arguments to dynamically insert values into the string. While the syntax may be slightly different, the functionality is essentially the same, making it easy for developers to transition between the two languages. By using the fmt.Sprintf() function, Go developers can easily format strings and create dynamic output for their applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |