The Java String format function is a method that allows you to create formatted strings by replacing placeholders with values. It takes a format string as its first argument, which contains placeholders for the values you want to insert. The placeholders are denoted by the percent sign followed by a letter that indicates the type of value to be inserted. For example, %s is used for strings, %d for integers, and %f for floating-point numbers. The method then takes additional arguments that correspond to the placeholders in the format string. These arguments are inserted into the string in the order they appear. The resulting string is returned by the method. Keep reading below to learn how to Java String format in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
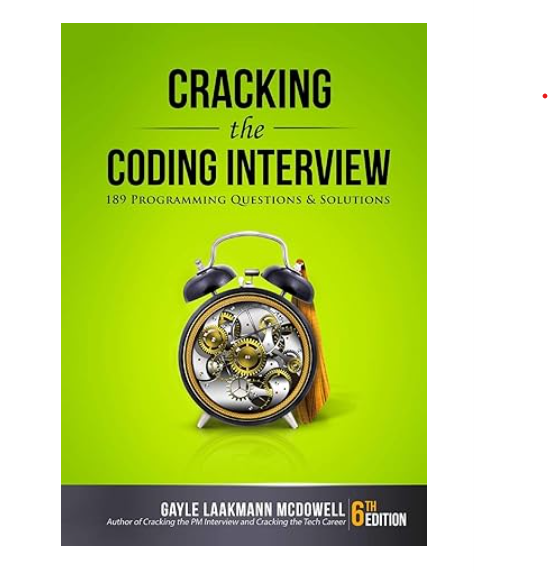
Java String format in Javascript With Example Code
Java String format is a powerful tool for formatting strings in Java. However, if you are working with JavaScript, you may be wondering how to achieve the same functionality. Fortunately, there are several ways to format strings in JavaScript that are similar to Java String format.
One way to format strings in JavaScript is to use the `replace()` method. This method allows you to replace parts of a string with other values. For example, if you have a string that contains the text “Hello, {0}!”, you can use the `replace()` method to replace the `{0}` placeholder with a value:
let name = "John";
let greeting = "Hello, {0}!";
let formattedGreeting = greeting.replace("{0}", name);
console.log(formattedGreeting); // Output: "Hello, John!"
Another way to format strings in JavaScript is to use template literals. Template literals are a new feature in ECMAScript 6 that allow you to embed expressions inside string literals. To use template literals, you enclose the string in backticks (`) instead of quotes:
let name = "John";
let greeting = `Hello, ${name}!`;
console.log(greeting); // Output: "Hello, John!"
In this example, the `${name}` expression is evaluated and the result is inserted into the string.
Finally, you can also use the `sprintf()` function from the sprintf-js library to format strings in JavaScript. This function works similarly to Java String format and allows you to specify placeholders and replacement values:
let name = "John";
let greeting = "Hello, %s!";
let formattedGreeting = sprintf(greeting, name);
console.log(formattedGreeting); // Output: "Hello, John!"
In conclusion, there are several ways to format strings in JavaScript that are similar to Java String format. Whether you choose to use the `replace()` method, template literals, or the `sprintf()` function, you can achieve powerful string formatting capabilities in your JavaScript code.
Equivalent of Java String format in Javascript
In conclusion, the equivalent function of Java’s String format in JavaScript is the template literal syntax. This feature allows developers to easily format strings by embedding expressions within backticks (`) and using placeholders (${expression}) to insert the values of those expressions. The template literal syntax is a powerful tool that can be used to create dynamic and readable strings in JavaScript. By using this feature, developers can avoid the need for complex string concatenation and formatting functions, making their code more concise and easier to maintain. Overall, the template literal syntax is a valuable addition to the JavaScript language and is a great alternative to Java’s String format function.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |