The Java String getBytes function is used to convert a string into a sequence of bytes. This function takes an optional parameter that specifies the character encoding to use for the conversion. If no encoding is specified, the default encoding of the platform is used. The resulting byte array can be used for various purposes, such as writing the string to a file or sending it over a network. It is important to note that the size of the resulting byte array may be larger than the length of the original string, as some characters may require multiple bytes to represent in certain encodings. Keep reading below to learn how to Java String getBytes in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
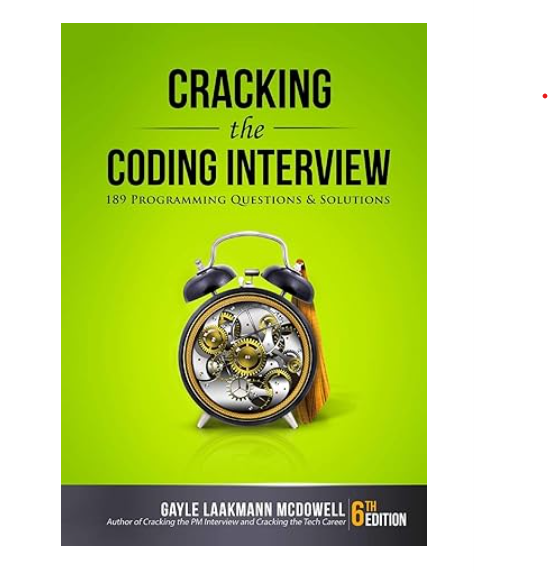
Java String getBytes in Go With Example Code
Java’s `String.getBytes()` method is a useful tool for converting a string into a byte array. However, if you’re working in Go, you may be wondering how to achieve the same functionality. In this blog post, we’ll explore how to use Go to replicate the `String.getBytes()` method in Java.
To start, let’s take a look at the `String.getBytes()` method in Java. This method takes an optional argument that specifies the character encoding to use when converting the string to bytes. If no encoding is specified, the default encoding for the platform is used.
Here’s an example of using `String.getBytes()` in Java:
String str = "Hello, world!";
byte[] bytes = str.getBytes();
This code will convert the string “Hello, world!” to a byte array using the default encoding.
Now, let’s see how we can achieve the same result in Go. Go provides a `[]byte` type that is similar to a byte array in Java. To convert a string to a `[]byte` in Go, we can use the `[]byte()` function.
Here’s an example of using `[]byte()` in Go:
str := "Hello, world!"
bytes := []byte(str)
This code will convert the string “Hello, world!” to a `[]byte` using the default encoding.
If you need to specify a different encoding, you can use the `encoding` package in Go. For example, to convert a string to a `[]byte` using the UTF-8 encoding, you can use the following code:
str := "Hello, world!"
bytes := []byte(str)
utf8Bytes, err := encoding.UTF8.Encode(bytes)
In this code, we first convert the string to a `[]byte` using the `[]byte()` function. We then use the `UTF8.Encode()` method from the `encoding` package to convert the `[]byte` to UTF-8 encoded bytes.
In conclusion, while Go doesn’t have a direct equivalent to Java’s `String.getBytes()` method, it’s easy to achieve the same functionality using the `[]byte()` function and the `encoding` package.
Equivalent of Java String getBytes in Go
In conclusion, the equivalent function of Java’s String getBytes() in Go is the built-in function called []byte(). This function converts a string into a slice of bytes, which can be used for various purposes such as encryption, encoding, and transmission over a network. While the syntax and usage of these functions may differ between Java and Go, the end result is the same. As a developer, it is important to understand the similarities and differences between programming languages and their functions, in order to effectively utilize them in your projects. By using the []byte() function in Go, you can easily convert strings into bytes and manipulate them as needed.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |