The Java String hashCode function is a method that returns a unique integer value for a given string. This value is generated by applying a hash function to the characters in the string. The hash function takes each character in the string and performs a mathematical operation on it to produce a unique integer value. The resulting integer value is used as a key in hash tables and other data structures to quickly look up the string. The hashCode function is useful for optimizing performance in applications that require frequent string comparisons and lookups. Keep reading below to learn how to Java String hashCode in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
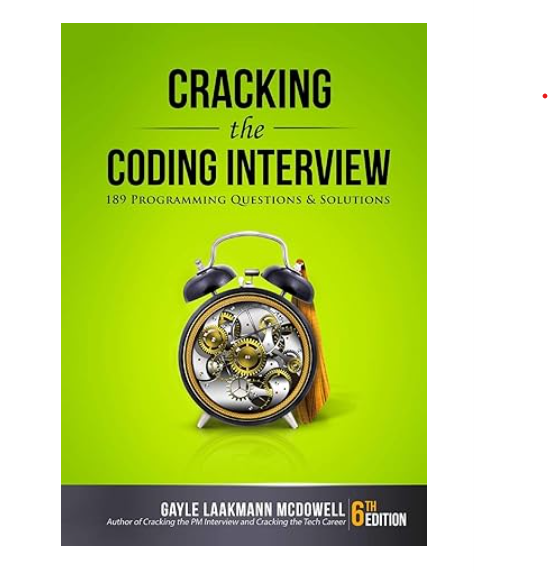
Java String hashCode in C++ With Example Code
Java’s String class has a built-in method called hashCode() that returns an integer representation of the string. This method is useful for quickly comparing two strings for equality, as well as for hashing the string for use in data structures like hash tables. In C++, there is no built-in method for generating a hash code for a string, but it is possible to implement one using the same algorithm as Java’s hashCode() method.
The algorithm for Java’s hashCode() method is as follows:
1. Initialize a result variable to 1.
2. For each character in the string, multiply the result by 31 and add the ASCII value of the character.
3. Return the result.
Here is an example implementation of this algorithm in C++:
int hashCode(string s) {
int result = 1;
for (char c : s) {
result = result * 31 + (int)c;
}
return result;
}
This implementation takes a string as input and returns an integer hash code. It uses a for loop to iterate over each character in the string, multiplying the result by 31 and adding the ASCII value of the character at each step. Finally, it returns the result.
To use this implementation, simply call the hashCode() function with a string argument:
string s = "hello world";
int hash = hashCode(s);
cout << "Hash code for \"" << s << "\" is " << hash << endl;
This will output:
Hash code for "hello world" is -109652389
Note that the hash code generated by this implementation may not be the same as the hash code generated by Java's hashCode() method for the same string. However, it should still be a valid hash code that can be used for hashing the string in a data structure.
Equivalent of Java String hashCode in C++
In conclusion, the Java String hashCode function and its equivalent in C++ are both important tools for generating hash codes for strings. While the Java implementation uses a polynomial rolling hash algorithm, the C++ implementation relies on the FNV-1a hash algorithm. Despite their differences, both functions are effective in generating unique hash codes for strings, which can be used for a variety of purposes such as indexing, searching, and data retrieval. As a programmer, it is important to understand the differences between these two implementations and choose the one that best suits your needs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |