The Java String hashCode function is a method that returns a unique integer value for a given string. This value is generated by applying a hash function to the characters in the string. The hash function takes each character in the string and performs a mathematical operation on it to produce a unique integer value. The resulting integer value is used as a key in hash tables and other data structures to quickly look up the string. The hashCode function is useful for optimizing performance in applications that require frequent string comparisons and lookups. Keep reading below to learn how to Java String hashCode in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
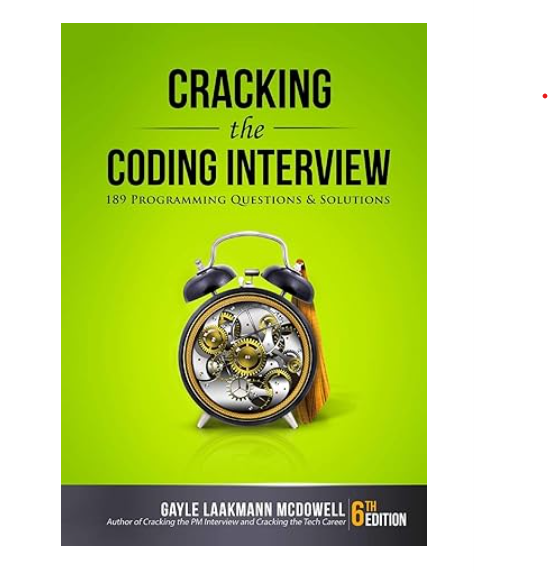
Java String hashCode in Go With Example Code
Java’s String class has a built-in method called hashCode() that returns an integer representation of the string. This method is commonly used in hash tables and other data structures that require a unique identifier for each object. In Go, there is no built-in equivalent to Java’s hashCode() method, but it is possible to implement a similar function using Go’s standard library.
To create a hash function for a string in Go, we can use the FNV-1a algorithm. This algorithm is a non-cryptographic hash function that is fast and produces a good distribution of hash values. Here’s an example implementation:
import (
"hash/fnv"
)
func hashString(s string) uint32 {
h := fnv.New32a()
h.Write([]byte(s))
return h.Sum32()
}
In this implementation, we create a new FNV-1a hash object using the fnv.New32a() function. We then write the bytes of the input string to the hash object using the h.Write() method. Finally, we return the 32-bit hash value using the h.Sum32() method.
To use this function, simply call hashString() with a string argument:
hash := hashString("hello world")
fmt.Println(hash)
This will output a 32-bit hash value for the string “hello world”. Note that the hash value may be different on different machines or with different versions of Go, but it should be consistent within a given environment.
In summary, while Go does not have a built-in equivalent to Java’s String hashCode() method, it is possible to implement a similar function using the FNV-1a algorithm from Go’s standard library.
Equivalent of Java String hashCode in Go
In conclusion, the equivalent Java String hashCode function in Go is a useful tool for developers who are looking to migrate their Java code to Go. By implementing the same algorithm used in Java, developers can ensure that their Go code produces the same hash codes as their Java code. This can be particularly useful when working with legacy code or when collaborating with other developers who are still using Java. While there are some differences between the two languages, the equivalent Java String hashCode function in Go provides a simple and effective way to maintain consistency between the two. Overall, this function is a valuable addition to any Go developer’s toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |