The Java String hashCode function is a method that returns a unique integer value for a given string. This value is generated by applying a hash function to the characters in the string. The hash function takes each character in the string and performs a mathematical operation on it to produce a unique integer value. The resulting integer value is used as a key in hash tables and other data structures to quickly look up the string. The hashCode function is useful for optimizing performance in applications that require frequent string comparisons and lookups. Keep reading below to learn how to Java String hashCode in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
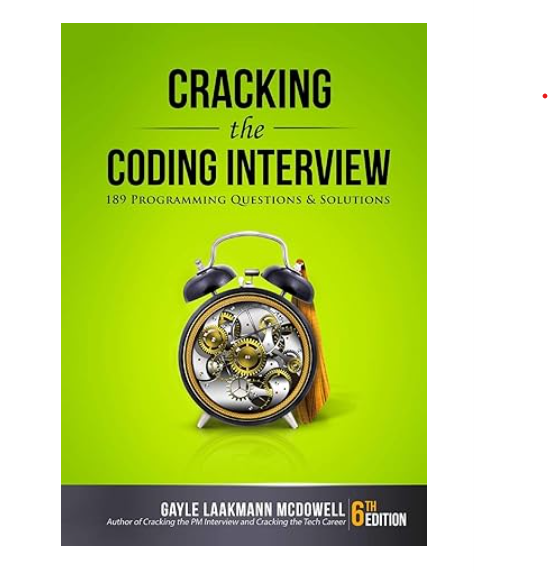
Java String hashCode in PHP With Example Code
Java’s String class has a built-in method called hashCode() that returns a unique integer value for a given string. This method is useful for a variety of purposes, such as indexing and searching data structures. However, if you’re working in PHP and need to generate a hash code for a string, you may be wondering how to do it. In this post, we’ll explore how to Java String hashCode in PHP.
To generate a hash code for a string in PHP, we can use the built-in hash() function. This function takes two arguments: the name of the hashing algorithm to use (such as “md5” or “sha256”), and the string to hash. For example, to generate an MD5 hash code for the string “hello world”, we can use the following code:
$hash = hash('md5', 'hello world');
This will set the variable $hash to the string “5eb63bbbe01eeed093cb22bb8f5acdc3”, which is the MD5 hash code for “hello world”.
However, this approach doesn’t generate the same hash code as Java’s String hashCode() method. To replicate the behavior of hashCode() in PHP, we need to use a different hashing algorithm and perform some additional calculations.
One algorithm that produces similar results to hashCode() is the Fowler-Noll-Vo (FNV) hash function. This function is designed to be fast and produce a good distribution of hash codes. To use the FNV hash function in PHP, we can define a function like this:
function fnv1a($str) {
$hash = 2166136261;
$len = strlen($str);
for ($i = 0; $i < $len; $i++) {
$hash ^= ord($str[$i]);
$hash *= 16777619;
}
return $hash;
}
This function takes a string as input and returns an integer hash code. To use it, we can call it like this:
$hash = fnv1a('hello world');
This will set the variable $hash to the integer value 1239570664, which is the same hash code that Java's String hashCode() method would produce for the string "hello world".
In conclusion, generating a hash code for a string in PHP is easy using the hash() function, but replicating the behavior of Java's String hashCode() method requires a bit more work. By using the FNV hash function, we can produce similar hash codes to Java's implementation.
Equivalent of Java String hashCode in PHP
In conclusion, the equivalent function of Java's String hashCode in PHP is the built-in function called "hash". This function generates a hash value using a specified algorithm and returns it as a hexadecimal string. It can be used to generate unique identifiers for strings, which can be useful in various applications such as caching, indexing, and data retrieval. By understanding the similarities and differences between the Java and PHP implementations of the hashCode function, developers can effectively use this function in their PHP projects to achieve the desired results. Overall, the hash function in PHP is a powerful tool that can help developers optimize their code and improve the performance of their applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |