The Java String hashCode function is a method that returns a unique integer value for a given string. This value is generated by applying a hash function to the characters in the string. The hash function takes each character in the string and performs a mathematical operation on it to produce a unique integer value. The resulting integer value is used as a key in hash tables and other data structures to quickly look up the string. The hashCode function is useful for optimizing performance in applications that require frequent string comparisons and lookups. Keep reading below to learn how to Java String hashCode in Python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
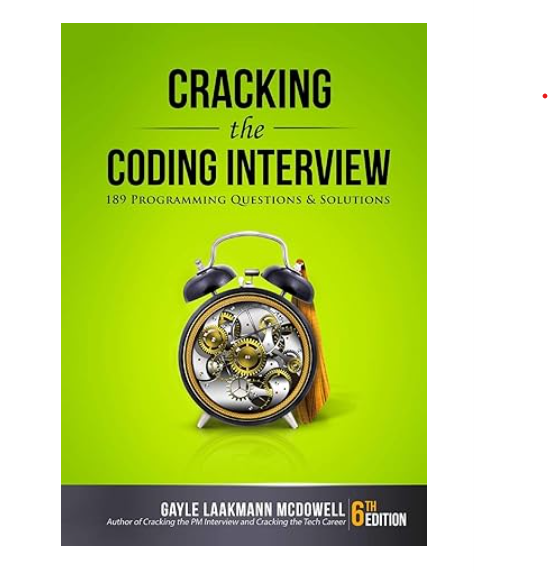
Java String hashCode in Python With Example Code
Java and Python are two popular programming languages used for various purposes. While Java is known for its robustness and performance, Python is known for its simplicity and ease of use. In this blog post, we will discuss how to implement the Java String hashCode method in Python.
The Java String hashCode method returns a hash code for the string. This hash code is generated based on the contents of the string. In Python, we can implement the same functionality using the built-in hash() function.
Here is an example code snippet that demonstrates how to implement the Java String hashCode method in Python:
def java_string_hashcode(s):
h = 0
for c in s:
h = (31 * h + ord(c)) & 0xFFFFFFFF
return ((h + 0x80000000) & 0xFFFFFFFF) - 0x80000000
In the above code, we define a function called java_string_hashcode that takes a string as input and returns its hash code. The function uses a similar algorithm as the Java String hashCode method to generate the hash code.
The algorithm works by iterating over each character in the string and multiplying the current hash value by 31 and adding the Unicode value of the character. The hash value is then masked with 0xFFFFFFFF to ensure that it fits within a 32-bit integer. Finally, the hash value is adjusted to be within the range of a signed 32-bit integer.
To use this function, simply call it with a string as input:
s = "Hello, world!"
hashcode = java_string_hashcode(s)
print(hashcode)
This will output the hash code for the string “Hello, world!”.
In conclusion, implementing the Java String hashCode method in Python is relatively straightforward using the built-in hash() function. By using a similar algorithm as the Java String hashCode method, we can generate hash codes for strings in Python that are compatible with Java hash codes.
Equivalent of Java String hashCode in Python
In conclusion, while Java and Python are two different programming languages, they both have similar functions that serve the same purpose. The Java String hashCode function and its equivalent in Python, the hash() function, both generate a unique integer value for a given string. However, it is important to note that the implementation of these functions may differ, and the resulting hash values may not be identical. As a Python developer, understanding the hash() function and its usage can be beneficial in various applications, including data structures and security. Overall, the equivalent Java String hashCode function in Python is the hash() function, and it is a powerful tool that can be used to generate unique hash values for strings in Python.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |