The Java String hashCode function is a method that returns a unique integer value for a given string. This value is generated by performing a mathematical calculation on the characters of the string. The calculation involves multiplying the ASCII value of each character by a prime number and adding them together. The resulting sum is then multiplied by another prime number and returned as the hash code. The hash code is used in various data structures, such as hash tables, to quickly locate and retrieve objects. It is important to note that while the hash code is unique for each string, collisions can occur where different strings produce the same hash code. Keep reading below to learn how to Java String hashCode in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
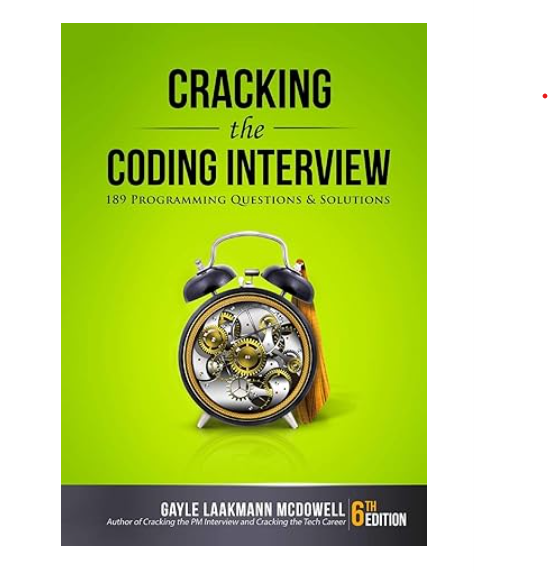
Java String hashCode in Rust With Example Code
Java’s `String` class has a method called `hashCode()` that returns an integer representation of the string. This method is commonly used in hashing algorithms and data structures. If you’re working in Rust and need to replicate this functionality, you can use the `std::collections::hash_map::DefaultHasher` struct.
To use `DefaultHasher`, you first need to create an instance of it:
use std::collections::hash_map::DefaultHasher;
use std::hash::{Hash, Hasher};
let mut hasher = DefaultHasher::new();
Next, you can call the `hash()` method on your string and pass in the `&mut hasher`:
let my_string = "hello world";
my_string.hash(&mut hasher);
let hash_value = hasher.finish() as i32;
The `hash_value` variable will now contain the integer representation of the string.
It’s important to note that the `DefaultHasher` struct is not guaranteed to produce the same hash value across different platforms or versions of Rust. If you need a consistent hash value, you should consider using a third-party hashing library.
Equivalent of Java String hashCode in Rust
In conclusion, the Rust programming language provides a similar implementation of the Java String hashCode function through its built-in hash function. While the two languages have different syntax and structures, the underlying concept of hashing remains the same. By using the Rust hash function, developers can efficiently generate hash codes for strings and other data types, which can be used for various purposes such as indexing, searching, and comparison. Overall, the Rust hash function is a powerful tool that can help developers write efficient and reliable code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |