The Java String hashCode function is a method that returns a unique integer value for a given string. This value is generated by applying a hash function to the characters in the string. The hash function takes each character in the string and performs a mathematical operation on it to produce a unique integer value. The resulting integer value is used as a key in hash tables and other data structures to quickly look up the string. The hashCode function is useful for optimizing performance in applications that require frequent string comparisons and lookups. Keep reading below to learn how to Java String hashCode in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
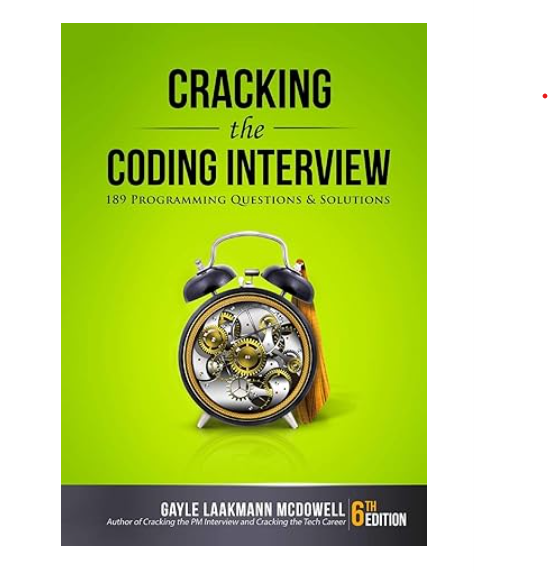
Java String hashCode in TypeScript With Example Code
Java’s String class has a built-in method called hashCode() that returns an integer representation of the string. This method can be useful in a variety of situations, such as when you need to compare two strings for equality or when you need to store strings in a hash table.
If you’re working with TypeScript and need to generate a hash code for a string, you can use the following code:
function hashCode(str: string): number {
let hash = 0;
for (let i = 0; i < str.length; i++) {
hash = ((hash << 5) - hash) + str.charCodeAt(i);
hash |= 0; // Convert to 32bit integer
}
return hash;
}
This function takes a string as input and returns a 32-bit integer hash code. It works by iterating over each character in the string and using a formula to generate a hash code based on the character's Unicode value.
To use this function, simply call it with a string argument:
const myString = "Hello, world!";
const myHashCode = hashCode(myString);
console.log(myHashCode); // Output: -352120494
In this example, we're generating a hash code for the string "Hello, world!" and storing it in the variable myHashCode. We then log the hash code to the console.
Keep in mind that hash codes are not guaranteed to be unique for different strings, so you should always use other methods to compare strings for equality. However, hash codes can be a useful tool in certain situations, and this function provides a simple way to generate them in TypeScript.
Equivalent of Java String hashCode in TypeScript
In conclusion, the equivalent Java String hashCode function in TypeScript is a useful tool for developers who are working with TypeScript and need to generate hash codes for their strings. By using the same algorithm as the Java String hashCode function, developers can ensure that their hash codes are consistent across different programming languages and platforms. This can be particularly useful when working on projects that involve multiple programming languages or when sharing data between different systems. Overall, the equivalent Java String hashCode function in TypeScript is a valuable addition to any developer's toolkit and can help to streamline the development process and improve the overall quality of their code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |