The Java String indexOf function is a method that returns the index of the first occurrence of a specified character or substring within a given string. It takes one or two arguments, the first being the character or substring to search for, and the second being an optional starting index from which to begin the search. If the character or substring is found, the method returns the index of its first occurrence within the string. If it is not found, the method returns -1. This function is useful for searching and manipulating strings in Java programs. Keep reading below to learn how to Java String indexOf in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
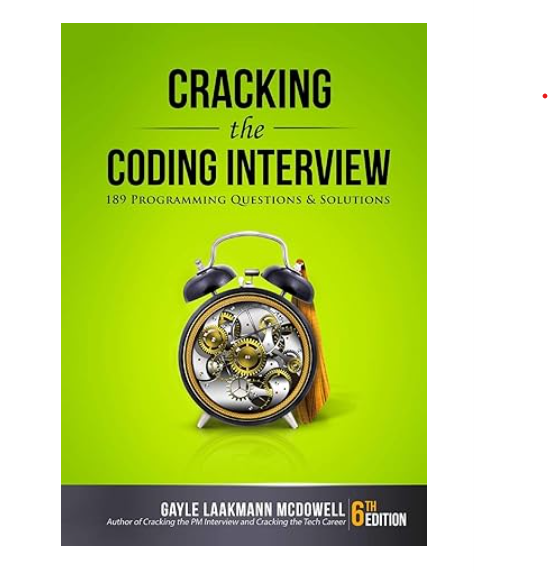
Java String indexOf in C# With Example Code
Java’s String class has a method called `indexOf` that returns the index of the first occurrence of a specified substring within the string. C# does not have a built-in method with the same name, but it does have a similar method called `IndexOf`.
To use `IndexOf` in C#, you can call it on a string object and pass in the substring you want to search for as an argument. The method will return the index of the first occurrence of the substring, or -1 if the substring is not found.
Here’s an example of how to use `IndexOf` in C#:
string myString = "Hello, world!";
int index = myString.IndexOf("world");
Console.WriteLine(index); // Output: 7
In this example, we create a string called `myString` and assign it the value “Hello, world!”. We then call the `IndexOf` method on `myString` and pass in the substring “world” as an argument. The method returns the index of the first occurrence of “world” in `myString`, which is 7.
If the substring is not found in the string, `IndexOf` will return -1. For example:
string myString = "Hello, world!";
int index = myString.IndexOf("foo");
Console.WriteLine(index); // Output: -1
In this example, we search for the substring “foo” in `myString`, which is not found. `IndexOf` returns -1 to indicate that the substring is not present in the string.
Overall, `IndexOf` in C# works similarly to Java’s `indexOf` method, and can be used to search for substrings within a string.
Equivalent of Java String indexOf in C#
In conclusion, the equivalent function of Java’s String indexOf in C# is the IndexOf method. This method is used to find the index of the first occurrence of a specified substring within a string. It works similarly to the Java function, taking in the substring as a parameter and returning the index of the first occurrence of the substring within the string. While the syntax and implementation may differ slightly between the two languages, the functionality remains the same. As a developer, it is important to be familiar with the various methods and functions available in different programming languages, and the IndexOf method in C# is a useful tool to have in your arsenal when working with strings.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |