The Java String indexOf function is a method that returns the index of the first occurrence of a specified character or substring within a given string. It takes one or two arguments, the first being the character or substring to search for, and the second being an optional starting index from which to begin the search. If the character or substring is found, the method returns the index of its first occurrence within the string. If it is not found, the method returns -1. This function is useful for searching and manipulating strings in Java programs. Keep reading below to learn how to Java String indexOf in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
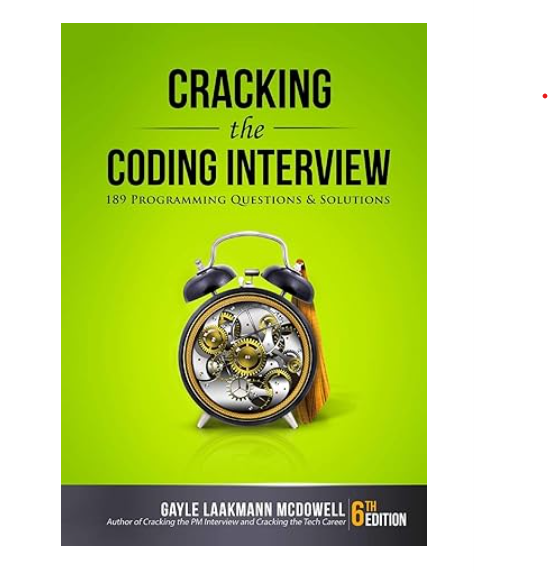
Java String indexOf in Rust With Example Code
Java developers who are transitioning to Rust may find themselves looking for the equivalent of the Java String `indexOf` method. In Rust, the equivalent method is `find`.
The `find` method returns an `Option
Here’s an example of how to use the `find` method in Rust:
let my_string = "hello world";
let index = my_string.find("world");
match index {
Some(i) => println!("Found world at index {}", i),
None => println!("Couldn't find world"),
}
In this example, we create a string `my_string` and then use the `find` method to search for the substring “world”. The `match` statement is used to handle the `Option
If you’re used to working with Java’s `indexOf` method, the `find` method in Rust may take some getting used to. However, with a little practice, you’ll find that it’s just as easy to use and can be just as powerful.
Equivalent of Java String indexOf in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to search for substrings within a string using the `find` method. This method is similar to the `indexOf` function in Java and allows developers to easily locate the position of a substring within a larger string. With its focus on performance and safety, Rust is a great choice for developers who want to build high-performance applications that are both reliable and secure. By leveraging the `find` method, Rust developers can easily search for substrings within their code and build robust applications that meet the needs of their users.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |