The Java String intern() function is used to return a canonical representation of a string. It returns a string that has the same contents as the original string but is guaranteed to be from a pool of unique strings. If the string already exists in the pool, then the intern() function returns a reference to that string. If the string does not exist in the pool, then it is added to the pool and a reference to the new string is returned. This function is useful for optimizing memory usage and improving performance in situations where many strings with the same content are created. Keep reading below to learn how to Java String intern in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
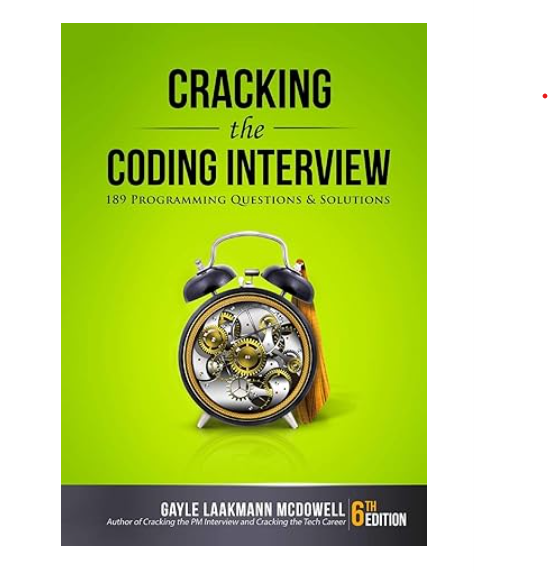
Java String intern in C# With Example Code
Java String intern is a method that returns a canonical representation for the string object. This means that if two string objects have the same value, they will have the same memory address. This can be useful for optimizing memory usage and improving performance. In C#, there is no direct equivalent to the Java String intern method, but there are ways to achieve similar functionality.
One way to achieve similar functionality is to use a string interning library, such as the one provided by the .NET framework. This library allows you to create a pool of unique strings, which can be used to reduce memory usage and improve performance. To use this library, you can call the String.Intern method, which will return a canonical representation of the string object.
Another way to achieve similar functionality is to use a custom string interning implementation. This can be done by creating a dictionary that maps string values to their canonical representations. Whenever a new string is created, you can check if it already exists in the dictionary. If it does, you can return the canonical representation. If it doesn’t, you can add it to the dictionary and return the new string object.
Here is an example of how to implement a custom string interning implementation in C#:
public class StringIntern
{
private static Dictionary
public static string Intern(string value)
{
if (_stringPool.TryGetValue(value, out string internedValue))
{
return internedValue;
}
else
{
_stringPool.Add(value, value);
return value;
}
}
}
To use this implementation, you can call the Intern method with a string value, which will return the canonical representation of the string object.
Equivalent of Java String intern in C#
In conclusion, the equivalent function to Java’s String intern() in C# is the String.Intern() method. This method allows for the creation of a single instance of a string in memory, which can be referenced by multiple variables. This can be useful in scenarios where memory usage is a concern, or when comparing strings for equality. By using String.Intern(), developers can optimize their code and improve performance. It’s important to note that while the functionality is similar, the syntax and implementation of the String.Intern() method in C# may differ slightly from the Java String intern() function. Nonetheless, both languages provide a way to optimize string handling and improve the efficiency of your code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |