The Java String intern() function is used to return a canonical representation of a string. It returns a string that has the same contents as the original string but is guaranteed to be from a pool of unique strings. If the string is already in the pool, then the intern() function returns a reference to that string. If the string is not in the pool, then it is added to the pool and a reference to the newly added string is returned. This function is useful for optimizing memory usage and improving performance in situations where many strings with the same content are created. Keep reading below to learn how to Java String intern in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
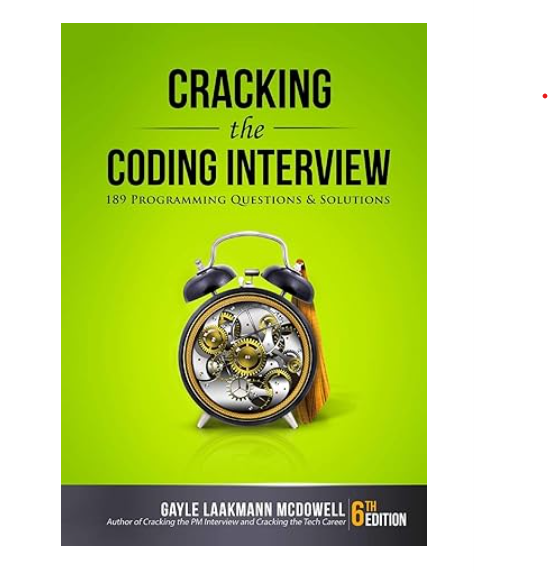
Java String intern in C++ With Example Code
Java String intern is a method that returns a canonical representation for the string object. This means that if two string objects have the same value, they will have the same memory address. This can be useful for optimizing memory usage and improving performance. In C++, we can achieve similar functionality using a combination of the std::string class and a hash table.
To implement string interning in C++, we can create a hash table that maps string values to their memory addresses. Whenever we create a new string object, we can check if it already exists in the hash table. If it does, we can return the existing memory address. If it doesn’t, we can create a new string object and add it to the hash table.
Here’s an example implementation:
#include
#include
std::unordered_map
const char* intern_string(const std::string& str) {
auto it = interned_strings.find(str);
if (it != interned_strings.end()) {
return it->second;
} else {
const char* interned_str = new char[str.size() + 1];
std::copy(str.begin(), str.end(), interned_str);
interned_str[str.size()] = '\0';
interned_strings[str] = interned_str;
return interned_str;
}
}
In this example, we use an unordered_map to store the interned strings. The keys are the string values, and the values are pointers to the memory addresses of the interned strings. The intern_string function takes a std::string as input and returns a const char* to the interned string.
To use this implementation, we can simply call the intern_string function with any string value:
const char* str1 = intern_string("hello");
const char* str2 = intern_string("world");
const char* str3 = intern_string("hello");
assert(str1 != str2);
assert(str1 == str3);
In this example, str1 and str3 will have the same memory address, while str2 will have a different memory address. This is because “hello” is interned and “world” is not.
Overall, implementing string interning in C++ can be a useful technique for optimizing memory usage and improving performance in certain situations.
Equivalent of Java String intern in C++
In conclusion, while Java’s String intern function is a useful tool for optimizing memory usage and improving performance, there is no direct equivalent in C++. However, there are several techniques that can be used to achieve similar results, such as using a hash table or a custom string pool implementation. Ultimately, the best approach will depend on the specific needs of your application and the trade-offs between memory usage and performance. By understanding the differences between Java and C++ and exploring the available options, developers can make informed decisions about how to manage strings in their code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |