The Java String intern() function is used to return a canonical representation of a string. It returns a string that has the same contents as the original string but is guaranteed to be from a pool of unique strings. If the string already exists in the pool, then the intern() function returns a reference to that string. If the string does not exist in the pool, then it is added to the pool and a reference to the new string is returned. This function is useful for optimizing memory usage and improving performance in situations where many strings with the same content are created. Keep reading below to learn how to Java String intern in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
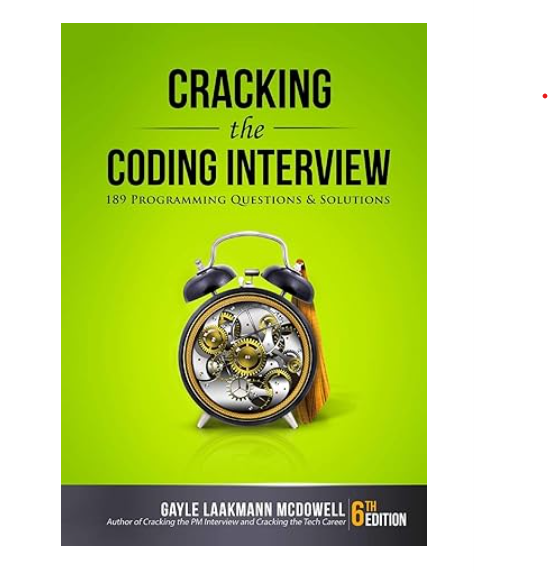
Java String intern in Go With Example Code
Java String intern is a method that returns a canonical representation of a string. This means that if two strings have the same value, they will be represented by the same object in memory. This can be useful for optimizing memory usage and improving performance.
In Go, there is no direct equivalent to Java’s String intern method. However, we can achieve similar functionality by using a map to store the canonical representations of strings.
Here’s an example implementation:
var stringPool = make(map[string]string)
func intern(s string) string {
if interned, ok := stringPool[s]; ok {
return interned
}
stringPool[s] = s
return s
}
In this implementation, we define a map called stringPool that will store the canonical representations of strings. The intern function takes a string as input and returns the canonical representation of that string.
The intern function first checks if the input string already exists in the stringPool map. If it does, the function returns the existing canonical representation. If not, the function adds the input string to the map and returns it.
To use the intern function, simply call it with a string as input:
s1 := "hello"
s2 := "hello"
s3 := "world"
interned1 := intern(s1)
interned2 := intern(s2)
interned3 := intern(s3)
fmt.Println(interned1 == interned2) // true
fmt.Println(interned1 == interned3) // false
In this example, s1 and s2 have the same value, so they should be represented by the same object in memory. The intern function returns the same canonical representation for both strings, so the comparison between interned1 and interned2 is true.
s3 has a different value, so it should be represented by a different object in memory. The intern function returns a different canonical representation for s3, so the comparison between interned1 and interned3 is false.
By using a map to store canonical representations of strings, we can achieve similar functionality to Java’s String intern method in Go.
Equivalent of Java String intern in Go
In conclusion, the equivalent function to Java’s String intern() in Go is the string.Intern() function. This function allows for the efficient use of memory by reusing existing strings instead of creating new ones. By using string.Intern(), Go developers can optimize their code and improve its performance. Additionally, this function can be particularly useful in scenarios where large amounts of string data are being processed, such as in web applications or data analysis. Overall, the string.Intern() function is a valuable tool for Go developers looking to optimize their code and improve its efficiency.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |