The Java String intern function is a method that returns a canonical representation of a string. It checks if the string already exists in the pool of strings and returns a reference to that string if it does. If the string does not exist in the pool, it adds it to the pool and returns a reference to the newly added string. This method is useful for conserving memory and improving performance in situations where the same string is used multiple times in an application. Keep reading below to learn how to Java String intern in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
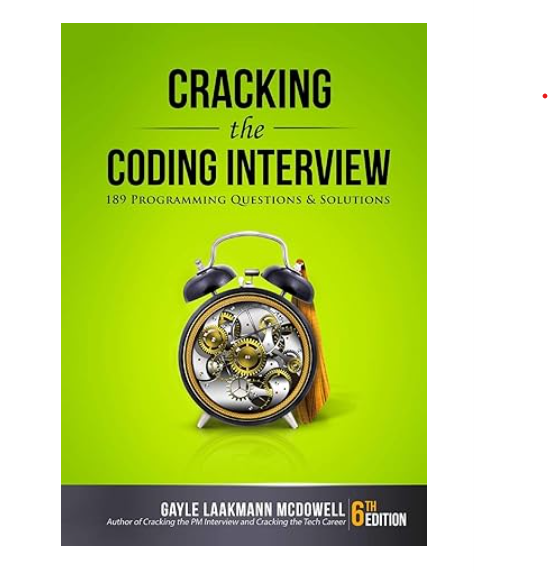
Java String intern in PHP With Example Code
Java String intern is a method that returns a canonical representation of a string. This means that it returns a reference to the same string object for all equal string values. This can be useful for optimizing memory usage and improving performance in certain situations.
In PHP, there is no direct equivalent to the Java String intern method. However, we can achieve similar functionality using PHP’s built-in string functions.
One way to emulate the Java String intern method in PHP is to use a static array to store references to unique string values. Here’s an example:
class StringIntern {
private static $strings = array();
public static function intern($string) {
if (!isset(self::$strings[$string])) {
self::$strings[$string] = $string;
}
return self::$strings[$string];
}
}
In this example, we define a class called StringIntern that contains a static array called $strings. The intern method takes a string as an argument and checks if it already exists in the $strings array. If it does, it returns the existing reference. If not, it adds the string to the array and returns the new reference.
To use this class, simply call the intern method with a string:
$string1 = StringIntern::intern("hello");
$string2 = StringIntern::intern("hello");
var_dump($string1 === $string2); // true
In this example, $string1 and $string2 both contain the string “hello”. However, because we used the StringIntern::intern method to create them, they both reference the same string object. This means that the comparison $string1 === $string2 returns true.
Overall, while PHP doesn’t have a built-in equivalent to the Java String intern method, we can use a static array and a custom class to achieve similar functionality.
Equivalent of Java String intern in PHP
In conclusion, the equivalent function of Java’s String intern() in PHP is the intern() function. This function is used to optimize memory usage by returning a single instance of a string if it already exists in memory. This can be particularly useful in situations where the same string is used multiple times throughout a program. By using the intern() function, you can reduce the amount of memory used by your PHP application and improve its overall performance. So, if you’re looking to optimize your PHP code and reduce memory usage, be sure to give the intern() function a try.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |