The Java String intern() function is used to return a canonical representation of a string. It returns a string that has the same contents as the original string but is guaranteed to be from a pool of unique strings. If the string already exists in the pool, then the intern() function returns a reference to that string. If the string does not exist in the pool, then it is added to the pool and a reference to the new string is returned. This function is useful for optimizing memory usage and improving performance in situations where many strings with the same content are created. Keep reading below to learn how to Java String intern in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
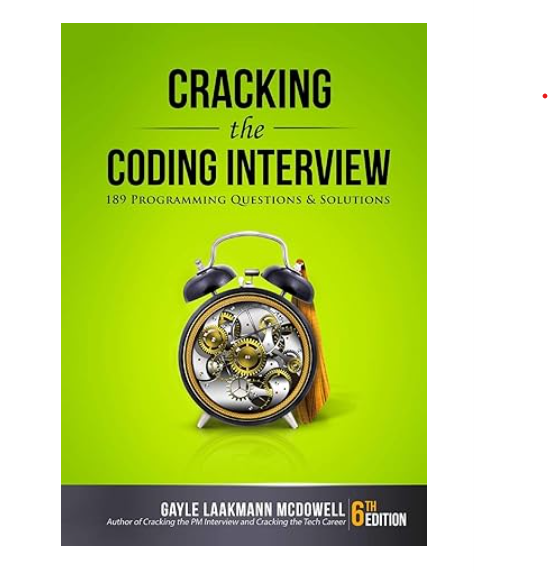
Java String intern in TypeScript With Example Code
Java String intern is a method that returns a canonical representation of a string. This means that if two strings have the same value, they will be represented by the same object in memory. This can be useful for optimizing memory usage and improving performance.
In TypeScript, there is no direct equivalent to the Java String intern method. However, you can achieve similar functionality by using a string pool.
A string pool is a data structure that stores a collection of unique strings. When you create a new string, you can check if it already exists in the pool. If it does, you can return the existing string object instead of creating a new one.
Here’s an example of how you can implement a string pool in TypeScript:
class StringPool {
private pool: { [key: string]: string } = {};
public intern(str: string): string {
if (this.pool[str]) {
return this.pool[str];
} else {
this.pool[str] = str;
return str;
}
}
}
In this example, the StringPool class has a private pool property that stores the unique strings. The intern method checks if the string already exists in the pool. If it does, it returns the existing string object. If not, it adds the string to the pool and returns the new string object.
To use the string pool, you can create a new instance of the StringPool class and call the intern method with your string:
const pool = new StringPool();
const str1 = pool.intern("hello");
const str2 = pool.intern("hello");
console.log(str1 === str2); // true
In this example, the intern method is called twice with the same string value. The first call creates a new string object and adds it to the pool. The second call returns the existing string object from the pool. The console.log statement outputs true, indicating that the two string objects are the same.
By using a string pool, you can achieve similar functionality to the Java String intern method in TypeScript. This can help you optimize memory usage and improve performance in your applications.
Equivalent of Java String intern in TypeScript
In conclusion, TypeScript provides a similar function to Java’s String intern method called “String literal types”. This feature allows developers to declare string values as types, which are then automatically interned by the TypeScript compiler. This can help improve performance and reduce memory usage in applications that heavily rely on string values. Additionally, TypeScript’s string literal types provide type safety and prevent common errors that can occur when working with strings in JavaScript. Overall, TypeScript’s string literal types are a powerful tool for developers looking to optimize their applications and write more robust code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |